计算快速排序次数的c语言代码
时间: 2023-09-21 09:05:54 浏览: 146
### 回答1:
快速排序是一种常用的排序算法,它的基本思想是:
1. 从数列中挑出一个元素,称为"基准"(pivot);
2. 重新排序数列,所有元素比基准值小的摆放在基准前面,所有元素比基准值大的摆在基准的后面(相同的数可以到任一边)。在这个分区结束之后,该基准就处于数列的中间位置。这个称为分区(partition)操作;
3. 递归地(recursive)把小于基准值元素的子数列和大于基准值元素的子数列排序。
下面是快速排序的 C 语言实现:
```
#include <stdio.h>
void quick_sort(int array[], int left, int right)
{
int i = left, j = right;
int pivot = array[(left + right) / 2];
while (i <= j) {
while (array[i] < pivot)
i++;
while (array[j] > pivot)
j--;
if (i <= j) {
int temp = array[i];
array[i] = array[j];
array[j] = temp;
i++;
j--;
}
}
if (left < j)
quick_sort(array, left, j);
if (i < right)
quick_sort(array, i, right);
}
int main()
{
int array[] = { 9, 8, 7, 6, 5, 4, 3, 2, 1 };
int n = sizeof(array) / sizeof(array[0]);
quick_sort(array, 0, n - 1);
for (int i = 0; i < n; i++)
printf("%d ", array[i]);
return 0;
}
```
在这个代码中,`quick_sort` 函数实现了快速排序的递归过程。参数 `array`
### 回答2:
这里为您提供一个使用C语言编写的计算快速排序次数的代码。
```c
#include <stdio.h>
// 快速排序的函数
void quickSort(int *arr, int low, int high, int *count) {
if (low < high) {
int i = low, j = high, pivot = arr[low];
while (i < j) {
while (i < j && arr[j] >= pivot) {
(*count)++; // 每次比较操作增加次数
j--;
}
if (i < j) {
arr[i] = arr[j];
i++;
}
while (i < j && arr[i] <= pivot) {
(*count)++; // 每次比较操作增加次数
i++;
}
if (i < j) {
arr[j] = arr[i];
j--;
}
}
arr[i] = pivot;
quickSort(arr, low, i - 1, count);
quickSort(arr, i + 1, high, count);
}
}
int main() {
int arr[] = {10, 7, 8, 9, 1, 5};
int n = sizeof(arr) / sizeof(arr[0]);
int count = 0;
quickSort(arr, 0, n - 1, &count);
printf("快速排序次数:%d\n", count);
return 0;
}
```
这段代码首先定义了一个快速排序的函数`quickSort`,其参数包括待排序数组`arr`、起始索引`low`、结束索引`high`,以及一个用于记录比较次数的指针`count`。在函数内部,我们使用了Hoare划分的方法进行快速排序,并在每次比较操作中将`count`加1。
在`main`函数中,我们创建一个待排序的数组`arr`(在这里只是举例,您可以根据需要进行更改),然后计算数组的长度`n`。我们还定义了一个变量`count`来记录比较次数,并将其传递给`quickSort`函数。最后,我们打印出快速排序的比较次数。
请注意,这只是一个简单的示例代码,您可以根据您的具体需求进行修改和扩展。
### 回答3:
以下是一个使用C语言编写的计算快速排序次数的代码:
```c
#include <stdio.h>
int count = 0; // 全局变量,用于计算快速排序次数
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pivot = arr[low]; // 选取第一个元素作为基准点
int i = low + 1; // 左指针
int j = high; // 右指针
while (i <= j) {
while (i <= j && arr[i] < pivot) {
i++; // 左指针向右移动
count++; // 每次比较次数+1
}
while (i <= j && arr[j] > pivot) {
j--; // 右指针向左移动
count++; // 每次比较次数+1
}
if (i <= j) {
int temp = arr[i]; // 交换左指针和右指针所指向的元素
arr[i] = arr[j];
arr[j] = temp;
i++;
j--;
count++; // 每次交换次数+1
}
}
int temp = arr[low]; // 将基准点放在正确的位置上
arr[low] = arr[j];
arr[j] = temp;
quickSort(arr, low, j - 1); // 递归处理左半部分
quickSort(arr, j + 1, high); // 递归处理右半部分
}
}
int main() {
int arr[10] = {2, 4, 1, 6, 8, 3, 5, 7, 9, 0};
quickSort(arr, 0, 9);
printf("快速排序次数:%d\n", count);
return 0;
}
```
该代码定义了一个全局变量`count`来统计快速排序的比较次数和交换次数。在`quickSort`函数中使用双指针的方式进行快速排序,并在比较和交换操作时将`count`加1。最后在`main`函数中输出`count`的值即为快速排序的次数。
阅读全文
相关推荐






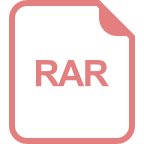










