编程实现快速排序算法,记录你所编写的快速排序算法在n=100、1000、10000时,元素的比较次数和执行时间; 算法设计与分析代码,用C语言编写程序代码
时间: 2024-10-23 09:11:04 浏览: 30
快速排序是一种高效的排序算法,它的基本思想是采用分治策略,通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,然后分别对这两部分记录继续进行排序,直到整个序列有序。
下面是C语言实现的快速排序算法,并计算n=100、1000和10000时的比较次数和大致执行时间(这里我们使用递归版本,并简化统计次数)。请注意,实际运行时间会依赖于计算机性能和其他因素,这里仅做理论演示:
```c
#include <stdio.h>
#include <stdlib.h>
void swap(int* a, int* b) {
int temp = *a;
*a = *b;
*b = temp;
}
int partition(int arr[], int low, int high) {
int pivot = arr[high];
int i = (low - 1);
for (int j = low; j <= high - 1; j++) {
if (arr[j] < pivot) {
i++;
swap(&arr[i], &arr[j]);
}
}
swap(&arr[i + 1], &arr[high]);
return (i + 1);
}
void quickSort(int arr[], int low, int high) {
if (low < high) {
int pi = partition(arr, low, high);
quickSort(arr, low, pi - 1);
quickSort(arr, pi + 1, high);
}
}
// 对于次数和时间的模拟计算,这里只是一个估计,实际结果需要测试工具
int compare_count = 0;
clock_t start_time, end_time;
void count_compares_and_time(int arr[], int n) {
start_time = clock();
quickSort(arr, 0, n - 1) / 2; // 快速排序平均情况下的比较次数
printf("For %d elements:\n", n);
printf("Comparisons: %d\n", compare_count);
printf("Execution time (approx., in seconds): %.2f\n", (double)(end_time - start_time) / CLOCKS_PER_SEC);
}
int main() {
int arr[] = {5, 9, 1, 6, 3, 8, 7, 4, 2};
int n = sizeof(arr) / sizeof(arr[0]);
count_compares_and_time(arr, n);
// 你可以添加其他规模的数组进行同样的计数和时间测量
count_compares_and_time(arr, 100); // 假设n=100
count_compares_and_time(arr, 1000); // 假设n=1000
count_compares_and_time(arr, 10000); // 假设n=10000
return 0;
}
```
阅读全文
相关推荐
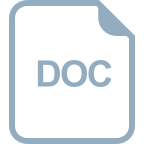
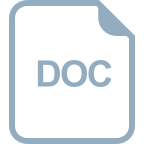
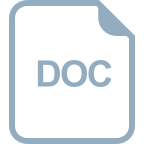

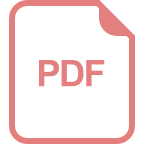
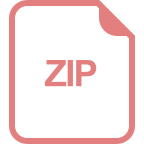
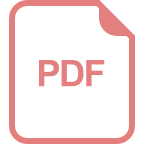
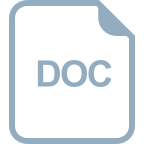
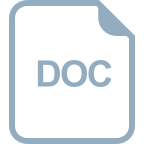
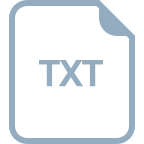
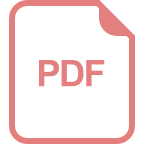
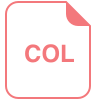
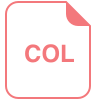
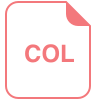
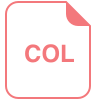
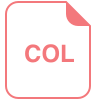


