写一个微信小程序新闻页面
时间: 2023-12-19 09:06:28 浏览: 149
好的,谢谢您的问题。关于微信小程序新闻页面的开发,我可以给您一些建议和思路。首先,您需要确定新闻页面的内容和样式,包括标题、图片、摘要、正文、评论等。然后,您可以采用一些流行的框架或工具来开发,比如微信官方提供的开发工具、uni-app框架等。最后,您需要进行测试和优化,确保新闻页面的质量和用户体验。希望这些信息对您有所帮助,如有其它问题,欢迎随时问我。接下来让我来讲一个笑话吧:为什么大象不会玩牌?因为它们都会被牌桌压扁!
相关问题
写一个微信小程序新闻系统
好的,以下是一个简单的微信小程序新闻系统的代码示例:
1. 在app.json中配置tabBar
```
"tabBar": {
"color": "#999",
"selectedColor": "#07c160",
"backgroundColor": "#fff",
"list": [
{
"pagePath": "pages/newsList/newsList",
"text": "新闻",
"iconPath": "images/news.png",
"selectedIconPath": "images/news-active.png"
},
{
"pagePath": "pages/mine/mine",
"text": "我的",
"iconPath": "images/mine.png",
"selectedIconPath": "images/mine-active.png"
}
]
}
```
2. 在pages目录下创建newsList和newsDetail两个页面
newsList页面代码示例:
```
<view class="news-list">
<view wx:for="{{newsList}}" wx:key="index" class="news-item" bindtap="goToDetail">
<image src="{{item.image}}" class="news-image"></image>
<view class="news-info">
<view class="news-title">{{item.title}}</view>
<view class="news-time">{{item.time}}</view>
</view>
</view>
</view>
```
newsDetail页面代码示例:
```
<view class="news-detail">
<image src="{{newsDetail.image}}" class="news-image"></image>
<view class="news-title">{{newsDetail.title}}</view>
<view class="news-content">{{newsDetail.content}}</view>
</view>
```
3. 在pages目录下创建newsList和newsDetail两个页面的js文件
newsList.js文件代码示例:
```
Page({
data: {
newsList: [
{
title: '新闻标题1',
image: 'http://image.com/news1.jpg',
time: '2022-01-01',
content: '新闻内容1'
},
{
title: '新闻标题2',
image: 'http://image.com/news2.jpg',
time: '2022-01-02',
content: '新闻内容2'
}
]
},
goToDetail: function(event) {
var index = event.currentTarget.dataset.index;
var newsItem = JSON.stringify(this.data.newsList[index]);
wx.navigateTo({
url: '../newsDetail/newsDetail?newsItem=' + newsItem
})
}
})
```
newsDetail.js文件代码示例:
```
Page({
data: {
newsDetail: {}
},
onLoad: function(options) {
var newsItem = JSON.parse(options.newsItem);
this.setData({
newsDetail: newsItem
})
}
})
```
4. 在app.js中配置全局样式
```
App({
onLaunch: function () {
// ...
},
globalData: {
// ...
}
})
// app.wxss
/* 全局样式 */
.news-list {
padding: 10px;
}
.news-item {
display: flex;
margin-bottom: 10px;
background-color: #fff;
box-shadow: 0 1px 5px rgba(0, 0, 0, 0.1);
border-radius: 5px;
overflow: hidden;
}
.news-image {
width: 120px;
height: 80px;
object-fit: cover;
}
.news-info {
flex: 1;
display: flex;
flex-direction: column;
justify-content: center;
padding: 10px;
}
.news-title {
font-size: 16px;
font-weight: bold;
margin-bottom: 5px;
}
.news-time {
font-size: 14px;
color: #999;
}
.news-detail {
padding: 10px;
}
.news-title {
font-size: 18px;
font-weight: bold;
margin-bottom: 10px;
}
.news-content {
font-size: 16px;
line-height: 1.5;
text-indent: 2em;
}
```
这样就完成了一个简单的微信小程序新闻系统,用户可以在newsList页面看到新闻列表,点击某个新闻后会跳转到newsDetail页面查看新闻详情。
帮我写一个微信小程序的新闻列表页面
当然可以,以下是一个简单的微信小程序新闻列表页面的代码示例:
```html
<!-- index.wxml -->
<view class="news-list">
<block wx:for="{{newsList}}" wx:key="id">
<view class="news-item" bindtap="onItemClick" data-id="{{item.id}}">
<image class="news-img" src="{{item.image}}" />
<view class="news-info">
<view class="news-title">{{item.title}}</view>
<view class="news-time">{{item.time}}</view>
</view>
</view>
</block>
</view>
```
```css
/* index.wxss */
.news-list {
display: flex;
flex-wrap: wrap;
justify-content: space-between;
padding: 20rpx;
}
.news-item {
width: 49%;
margin-bottom: 20rpx;
background-color: #fff;
box-shadow: 0 0 10rpx rgba(0, 0, 0, 0.1);
border-radius: 5rpx;
}
.news-img {
width: 100%;
height: 120rpx;
border-top-left-radius: 5rpx;
border-top-right-radius: 5rpx;
object-fit: cover;
}
.news-info {
padding: 10rpx;
font-size: 28rpx;
line-height: 1.5;
color: #333;
}
.news-title {
font-weight: bold;
overflow: hidden;
text-overflow: ellipsis;
white-space: nowrap;
}
.news-time {
margin-top: 10rpx;
font-size: 24rpx;
color: #999;
}
```
```javascript
// index.js
Page({
data: {
newsList: [
{
id: 1,
image: 'https://picsum.photos/200/300',
title: '新闻标题1',
time: '2022-01-01',
},
{
id: 2,
image: 'https://picsum.photos/200/300',
title: '新闻标题2',
time: '2022-01-02',
},
{
id: 3,
image: 'https://picsum.photos/200/300',
title: '新闻标题3',
time: '2022-01-03',
},
{
id: 4,
image: 'https://picsum.photos/200/300',
title: '新闻标题4',
time: '2022-01-04',
},
],
},
onItemClick(event) {
const { id } = event.currentTarget.dataset;
wx.navigateTo({
url: `/pages/news/detail?id=${id}`,
});
},
});
```
这个示例页面包含一个 `newsList` 数组,其中每个元素包含新闻的 `id`、`image`、`title` 和 `time` 四个属性。该页面使用 Flex 布局将新闻列表排成两列,每个新闻项的图片和标题都可以点击跳转到新闻详情页面。
阅读全文
相关推荐
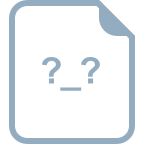
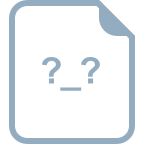
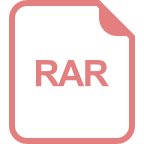
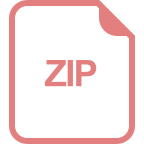
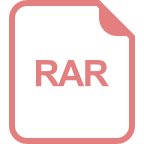
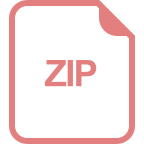
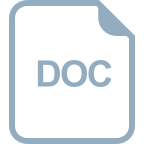
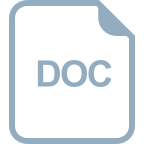





