自动浇花代码
时间: 2023-07-09 22:05:52 浏览: 149
以下是一个简单的自动浇花代码示例:
```python
import RPi.GPIO as GPIO
import time
# 设置GPIO模式为BCM
GPIO.setmode(GPIO.BCM)
# 设置GPIO口
GPIO.setup(18, GPIO.OUT)
# 打开GPIO口
GPIO.output(18, GPIO.HIGH)
# 等待5秒钟
time.sleep(5)
# 关闭GPIO口
GPIO.output(18, GPIO.LOW)
# 清理GPIO口
GPIO.cleanup()
```
这个示例代码使用树莓派的GPIO口来控制电磁阀开关,通过设置GPIO口为输出模式,打开GPIO口来开启电磁阀,等待一段时间后关闭GPIO口来关闭电磁阀。
相关问题
esp32 自动浇花系统的米思琪代码
ESP32自动浇花系统通常会利用物联网技术,结合Arduino或Espressif平台编写代码,通过WiFi连接远程控制设备,定时开关水阀来进行浇水。以下是一个简化的米思琪(MiSoul)风格的ESP32自动浇花系统代码示例:
```cpp
#include <ESP8266WiFi.h>
#include <WiFiClientSecure.h>
#include <DNSServer.h>
// WiFi设置
const char* ssid = "your_SSID";
const char* password = "your_PASSWORD";
// 云端服务器信息
const char* mqtt_server = "mqtt.your_server.com";
const int mqtt_port = 1883;
const char* mqtt_topic = "home/garden/sprinkler";
// 定义定时任务间隔
const unsigned long watering_interval = 60 * 60 * 2; // 每两小时浇水一次
DNSServer dnsServer;
WiFiClientSecure client;
void setup() {
Serial.begin(115200);
if (!WiFi.begin(ssid, password)) {
Serial.println("Connection failed!");
return;
}
while (WiFi.status() != WL_CONNECTED) {
delay(1000);
Serial.print(".");
}
Serial.println();
if (!client.connect("ESPClient", mqtt_server, mqtt_port)) {
Serial.println("MQTT connection failed!");
return;
}
// 注册DNS解析服务
dnsServer.setDomain("your_domain");
}
void loop() {
// 连接并发布主题到MQTT服务器
if (dnsServer.processNextQuery(client)) {
String host = dnsServer.getHostByName(dnsServer queries[0].name);
WiFiUDP udp;
udp.begin(host.c_str(), 1883);
String message = "sprinkle_start";
WiFiClientSecure secureClient(udp);
if (secureClient.connected()) {
secureClient.publish(mqtt_topic, message);
} else {
Serial.println("Publishing failed.");
}
} else {
delay(1000); // 如果DNS查询失败,等待一秒钟再试
}
// 按照预定时间间隔浇水
static unsigned long lastWatered = 0;
if (millis() - lastWatered > watering_interval) {
lastWatered = millis();
publishSprinklerStatus("watering"); // 根据实际硬件操作编写这个函数
}
delay(1000); // 程序主循环休眠时间
}
void publishSprinklerStatus(String status) {
// 发布喷水状态到MQTT主题
// 这里可以添加具体的代码来发送状态更新,例如:
// secureClient.publish(mqtt_topic + "/status", status.c_str());
}
```
基于单片机的自动浇花灌溉系统设计代码以及解释
基于单片机(如Arduino或51系列)的自动浇花灌溉系统设计通常包括硬件部分和软件控制部分。这里简化地给出一个基本流程:
**硬件部分:**
1. **传感器:** 水分传感器(如电容式湿度传感器),检测土壤湿度。
2. **继电器:** 控制水泵开启和关闭,连接到水源。
3. **定时器:** 单片机上集成的定时器用于设定灌溉周期。
4. **电源管理:** 单稳态触发器或外部电源模块提供稳定的电压。
**软件部分(C语言示例,假设使用Arduino):**
```c
#include <Servo.h>
#define PUMP_PIN 9 // 继电器连接的数字IO口
#define SENSOR_PIN A0 // 湿度传感器连接的模拟IO口
Servo pump; // 创建继电器驱动器实例
int sensorValue = 0;
void setup() {
Serial.begin(9600); // 开启串行通信
pinMode(PUMP_PIN, OUTPUT); // 设置继电器输出模式
pump.attach(PUMP_PIN); // 初始化继电器
}
void loop() {
sensorValue = analogRead(SENSOR_PIN); // 读取土壤湿度值
if (sensorValue < THRESHOLD) { // 当湿度低于预设阈值
Serial.println("Soil is dry, starting irrigation...");
pump.write(180); // 继电器打开,开始浇水
delay(IRRIGATION_DELAY); // 等待一段时间
pump.write(0); // 继电器关闭,停止浇水
} else {
Serial.println("Soil is moist enough.");
}
delay(SAMPLE_RATE); // 定时采集数据
}
```
阅读全文
相关推荐
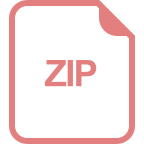
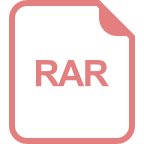
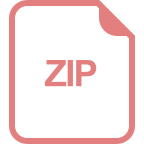
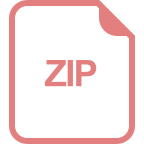
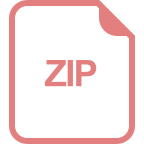
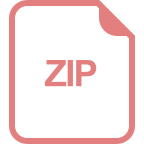
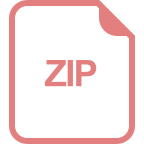





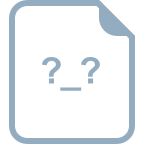