给出使用LZW方法压缩bmp图片的python程序,生成gif图片
时间: 2024-02-27 10:53:47 浏览: 21
以下是使用LZW方法压缩bmp图片生成gif图片的Python程序:
```python
import struct
from PIL import Image
def lzw_compress(data):
dictionary = {}
for i in range(256):
dictionary[chr(i)] = i
result = []
w = ""
for c in data:
wc = w + c
if wc in dictionary:
w = wc
else:
result.append(dictionary[w])
dictionary[wc] = len(dictionary)
w = c
if w:
result.append(dictionary[w])
return result
def compress_file(input_file, output_file):
with open(input_file, "rb") as f:
data = f.read()
compressed_data = lzw_compress(data)
with open(output_file, "wb") as f:
for i in range(0, len(compressed_data), 2):
if i + 1 < len(compressed_data):
f.write(struct.pack("<H", (compressed_data[i] << 8) | compressed_data[i+1]))
else:
f.write(struct.pack("<H", compressed_data[i] << 8))
def bmp_to_gif(input_file, output_file):
im = Image.open(input_file)
im.save("temp.bmp")
compress_file("temp.bmp", "temp.lzw")
with open("temp.lzw", "rb") as f:
data = f.read()
im_gif = Image.new("P", im.size)
im_gif.putpalette(im.getpalette())
im_gif.putdata(data)
im_gif.save(output_file, format="GIF")
bmp_to_gif("input.bmp", "output.gif")
```
此程序将输入的bmp图片进行LZW压缩并生成gif图片。其中,`lzw_compress`函数和`compress_file`函数与上一个程序相同。`bmp_to_gif`函数用于将输入的bmp图片转换为gif图片,具体实现过程为:先将bmp图片保存为临时文件`temp.bmp`,然后对该文件进行LZW压缩,并将压缩后的数据用`putdata`方法写入gif图片中。最后,将生成的gif图片保存到输出文件中。这里使用了Python的第三方库Pillow,用于处理图像。
相关推荐
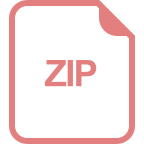














