阅读程序,输出结果 #include <stdio.h> #include <string.h> int main() { const char *s= "Hello"; printf("%d", strlen(s)); return 0; }
时间: 2024-03-28 18:41:18 浏览: 84
这个程序的输出结果是:
5
这个程序定义了一个字符串指针 `s` 并且初始化为字符串常量 "Hello" 的地址。然后使用了 `strlen()` 函数来获取该字符串的长度,并将结果打印到终端上。由于字符串 "Hello" 中包含了 5 个字符,因此 `strlen()` 函数返回值为 5,程序最终输出 5。
相关问题
#include<stdio.h> #include<ctype.h> #include<stdlib.h> #include<string.h> char *str(char *name){ char *s1="hello "; strcat(s1,name); return s1; } int main(){ char*name; name=(char*)malloc(20); printf("输入你的名字:"); gets(name); printf("%s",str(name)); }如何修改代码
在您提供的代码中,存在几个需要进行修改的地方。首先,`gets()` 函数是不安全的,已被废弃,应该使用更安全的替代函数 `fgets()`。其次,在 `str()` 函数中,您将字符串常量 `s1` 的地址返回,这是不安全的,因为 `s1` 是一个局部变量,函数结束后会被销毁。下面是修改后的代码:
```c
#include <stdio.h>
#include <ctype.h>
#include <stdlib.h>
#include <string.h>
char* str(const char* name) {
char* s1 = malloc(strlen(name) + 7); // 为新字符串分配足够的内存
strcpy(s1, "hello ");
strcat(s1, name);
return s1;
}
int main() {
char name[20];
printf("输入你的名字: ");
fgets(name, sizeof(name), stdin); // 使用fgets()获取用户输入
name[strcspn(name, "\n")] = '\0'; // 去除输入字符串中的换行符
printf("%s", str(name));
free(str(name)); // 释放通过malloc分配的内存
return 0;
}
```
这样修改后的代码更安全,避免了不安全的函数和内存问题。同时,我们也对用户输入的字符串进行了处理,去除了可能存在的换行符。请注意在使用完成后释放通过 `malloc` 分配的内存,以避免内存泄漏。
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <stdint.h> #define SHA1_BLOCK_SIZE 20 #define SHA1_DIGEST_SIZE 5
SHA-1是一种密码散列函数,用于产生消息的数字指纹。SHA-1算法的输出长度为160位,即20个字节。在C语言中,可以使用以下代码来实现SHA-1算法:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#define SHA1_BLOCK_SIZE 20
#define SHA1_DIGEST_SIZE 5
void sha1(const uint8_t *message, size_t message_len, uint8_t *digest) {
// TODO: 实现SHA-1算法
}
int main() {
uint8_t message[] = "hello world";
uint8_t digest[SHA1_DIGEST_SIZE];
sha1(message, strlen((char *)message), digest);
printf("SHA-1 digest: ");
for (int i = 0; i < SHA1_DIGEST_SIZE; i++) {
printf("%02x", digest[i]);
}
printf("\n");
return 0;
}
```
阅读全文
相关推荐
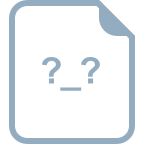
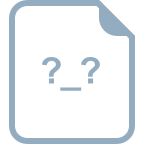














