有两2*3规模的矩阵a和b,编写程序,求两个矩阵之和。重做插入运算符和提取运算符,使之能用于该矩阵的输入和输出。重载运算符“+”,使之能用于矩阵相加,如c=a+b.
时间: 2024-05-02 17:17:32 浏览: 84
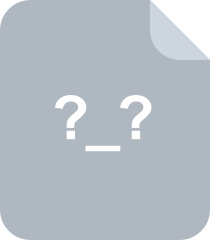
C语言程序设计-编写程序, 求矩阵(3行3列)与2的乘积 .c

下面是使用C++实现的示例代码:
```cpp
#include <iostream>
using namespace std;
class Matrix {
private:
int row, col; // 矩阵的行数和列数
int **data; // 指向矩阵数据的指针
public:
Matrix(int r, int c) : row(r), col(c) {
data = new int*[row];
for (int i = 0; i < row; i++) {
data[i] = new int[col];
}
}
~Matrix() {
for (int i = 0; i < row; i++) {
delete[] data[i];
}
delete[] data;
}
// 重载插入运算符
friend istream& operator>>(istream& in, Matrix& m) {
for (int i = 0; i < m.row; i++) {
for (int j = 0; j < m.col; j++) {
in >> m.data[i][j];
}
}
return in;
}
// 重载提取运算符
friend ostream& operator<<(ostream& out, const Matrix& m) {
for (int i = 0; i < m.row; i++) {
for (int j = 0; j < m.col; j++) {
out << m.data[i][j] << " ";
}
out << endl;
}
return out;
}
// 重载加号运算符
Matrix operator+(const Matrix& other) const {
Matrix result(row, col);
for (int i = 0; i < row; i++) {
for (int j = 0; j < col; j++) {
result.data[i][j] = data[i][j] + other.data[i][j];
}
}
return result;
}
};
int main() {
Matrix a(2, 3);
Matrix b(2, 3);
cout << "请输入矩阵A的元素:" << endl;
cin >> a;
cout << "请输入矩阵B的元素:" << endl;
cin >> b;
Matrix c = a + b;
cout << "矩阵A加B的结果为:" << endl;
cout << c;
return 0;
}
```
在程序中,我们定义了一个 `Matrix` 类来表示矩阵,并实现了矩阵的输入、输出和加法运算。在 `Matrix` 类中,我们使用了一个二维数组来存储矩阵的数据。在构造函数中,我们使用了动态内存分配来分配二维数组的内存空间。在析构函数中,我们释放了动态分配的内存空间,避免了内存泄漏。
在 `Matrix` 类中,我们重载了插入运算符 `>>` 和提取运算符 `<<`,使之能够用于矩阵的输入和输出。在重载运算符时,我们使用了 `friend` 关键字,将运算符函数声明为友元函数,以便访问类的私有成员变量。
在 `Matrix` 类中,我们还重载了加号运算符 `+`,使之能够用于矩阵相加。在重载加号运算符时,我们定义了一个新的矩阵 `result`,并将 `result` 的每个元素初始化为两个矩阵对应元素之和。最后返回 `result`,得到矩阵相加的结果。
在 `main()` 函数中,我们创建了两个 2*3 大小的矩阵 `a` 和 `b`,并使用插入运算符 `>>` 从标准输入流中读取矩阵的元素。接着,我们使用加号运算符 `+` 将矩阵 `a` 和 `b` 相加,得到矩阵相加的结果 `c`。最后,我们使用提取运算符 `<<` 将结果输出到标准输出流中。
阅读全文
相关推荐
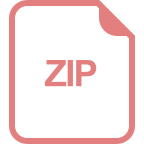
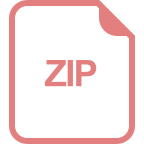
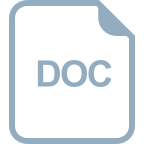
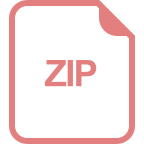
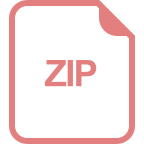
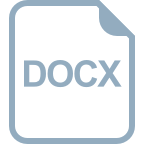
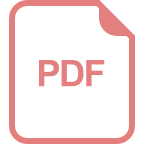
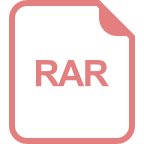
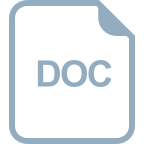
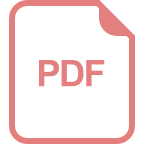
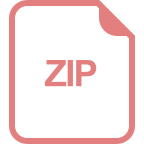
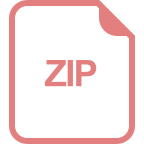