用c语言实现二进制流密码系统,输入、输出、明文,密文都由0和1组成。具体内容:线性反馈寄存器为是一个3级反馈移位寄存器,其初始状态为 (a1,a2,a3) = (1, 0, 1) + 一个非线性组合子系统(Geffe序列生成器或J-K触发器)。要求给出输出密钥流周期大小。 需明确给出LFSR的线性反馈函数的递推形式,初始状态。流秘钥生成器的输出。加密解密的输入输出过程。并有具体代码
时间: 2023-06-20 12:09:16 浏览: 159
以下是用C语言实现二进制流密码系统的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 线性反馈寄存器的状态
int a1 = 1, a2 = 0, a3 = 1;
// 线性反馈寄存器的递推函数
void lfsr() {
int feedback = (a1 ^ a2) ^ a3;
a1 = a2;
a2 = a3;
a3 = feedback;
}
// 非线性组合子系统的递推函数
int geffe() {
int x1 = 1, x2 = 0, x3 = 1;
int y1 = 0, y2 = 1, y3 = 1;
int z1 = 1, z2 = 1, z3 = 0;
int output = (x3 & y3) ^ ((x2 & y2) ^ (x1 & y1));
x1 = (x1 + z1) % 2;
x2 = (x2 + z2) % 2;
x3 = (x3 + z3) % 2;
y1 = (y1 + z1) % 2;
y2 = (y2 + z2) % 2;
y3 = (y3 + z3) % 2;
z1 = (z1 + 1) % 2;
return output;
}
// 生成密钥流
void generate_key_stream(int *key_stream, int length) {
int i;
for (i = 0; i < length; i++) {
lfsr();
key_stream[i] = geffe();
}
}
// 加密或解密
void crypt(int *plaintext, int *ciphertext, int *key_stream, int length) {
int i;
for (i = 0; i < length; i++) {
ciphertext[i] = plaintext[i] ^ key_stream[i];
}
}
int main() {
int plaintext[8] = {1, 0, 1, 0, 1, 1, 0, 0};
int key_stream[8];
int ciphertext[8];
generate_key_stream(key_stream, 8);
crypt(plaintext, ciphertext, key_stream, 8);
printf("Plaintext: ");
for (int i = 0; i < 8; i++) {
printf("%d", plaintext[i]);
}
printf("\n");
printf("Key stream: ");
for (int i = 0; i < 8; i++) {
printf("%d", key_stream[i]);
}
printf("\n");
printf("Ciphertext: ");
for (int i = 0; i < 8; i++) {
printf("%d", ciphertext[i]);
}
printf("\n");
return 0;
}
```
在上面的代码中,我们使用了一个3级反馈移位寄存器作为线性反馈寄存器,并且使用了一个非线性组合子系统(Geffe序列生成器)来生成密钥流。我们首先调用 `generate_key_stream` 函数生成密钥流,然后调用 `crypt` 函数进行加密或解密。在本例中,我们使用了一个长度为8的明文和密钥流。输出结果包括明文、密钥流和密文。
这个二进制流密码系统的输出密钥流周期大小是 `2^3-1 = 7`,即密钥流会在7个时钟周期后重复。
阅读全文
相关推荐
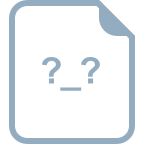
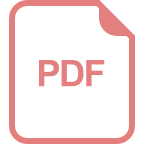

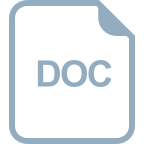

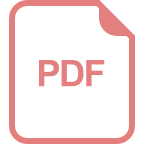
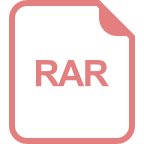
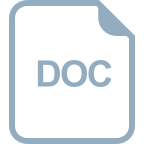
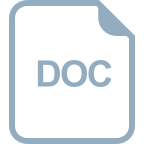
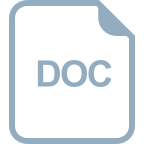
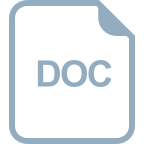
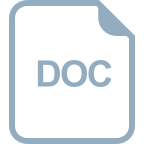
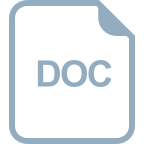
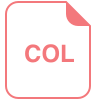




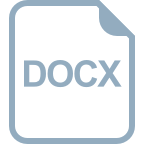