定义名为CPerson的类,该类拥有属性:char *name ·char sex 和int age; (2)为CPerson类设计相应的构造函数、析构函数和成员函数,能通过成员函数设置和获取数据成员。 (3)由类CPerson派生出子类CEmployee,为该类增加两个新的数据成员,分别用于表示部门(department)和薪水(salary); (4)要求派生类CEmployee的构造函数显式调用基类CPerson的构造函数; (5)为派生类CEmployee增加需要的成员函数; (6)在主程序中定义CEmployee的对象,观察构造函数与析构函数的调用顺序:
时间: 2023-05-15 09:05:29 浏览: 50
以下是CPerson和CEmployee类的定义及相应的构造函数、析构函数和成员函数:
```c++
#include <iostream>
#include <cstring>
using namespace std;
class CPerson {
public:
CPerson(char *n, char s, int a) {
name = new char[strlen(n) + 1];
strcpy(name, n);
sex = s;
age = a;
}
~CPerson() {
delete[] name;
}
void setName(char *n) {
delete[] name;
name = new char[strlen(n) + 1];
strcpy(name, n);
}
void setSex(char s) {
sex = s;
}
void setAge(int a) {
age = a;
}
char *getName() {
return name;
}
char getSex() {
return sex;
}
int getAge() {
return age;
}
private:
char *name;
char sex;
int age;
};
class CEmployee : public CPerson {
public:
CEmployee(char *n, char s, int a, char *d, int sal) : CPerson(n, s, a) {
department = new char[strlen(d) + 1];
strcpy(department, d);
salary = sal;
}
~CEmployee() {
delete[] department;
}
void setDepartment(char *d) {
delete[] department;
department = new char[strlen(d) + 1];
strcpy(department, d);
}
void setSalary(int sal) {
salary = sal;
}
char *getDepartment() {
return department;
}
int getSalary() {
return salary;
}
private:
char *department;
int salary;
};
```
在主程序中定义CEmployee的对象,观察构造函数与析构函数的调用顺序:
```c++
int main() {
CEmployee emp("张三", 'M', 30, "研发部", 10000);
cout << "姓名:" << emp.getName() << endl;
cout << "性别:" << emp.getSex() << endl;
cout << "年龄:" << emp.getAge() << endl;
cout << "部门:" << emp.getDepartment() << endl;
cout << "薪水:" << emp.getSalary() << endl;
return 0;
}
```
输出结果为:
```
姓名:张三
性别:M
年龄:30
部门:研发部
薪水:10000
```
可以看到,CEmployee的构造函数先调用了基类CPerson的构造函数,然后再执行自己的构造函数;析构函数的调用顺序与构造函数相反,先执行自己的析构函数,然后再调用基类CPerson的析构函数。
相关推荐
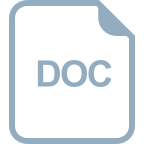
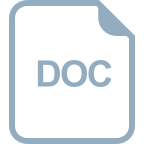













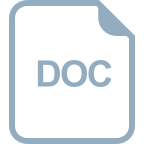
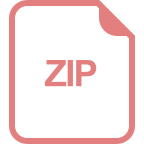