def __iter__(self): self.count = 0 return self详细解释一下这段代码
时间: 2024-02-16 14:46:48 浏览: 27
This code defines an iterator for a class.
The `__iter__` method is a special method in Python that returns an iterator object. In this case, the method initializes a count variable to 0 and returns the object itself, which is an instance of the class.
When the object is used in a `for` loop, the `__next__` method is called on the iterator object, which returns the next value in the sequence. In this case, the `__next__` method increments the count variable and returns it.
So, when you use the iterator in a `for` loop, it will generate an infinite sequence of integers starting from 0.
相关问题
class FibIterator: count = 0 now = 1 #初始值分别设置为1和0,方便__next__函数处理 last = 0 # def __init__(self,count): self.count = count def __iter__(self): return self def __next__(self): #********** Begin *********# #********** End **********# def GetFib(count): return FibIterator(count)
这段代码是一个迭代器,用于生成斐波那契数列,其实现方式为:
1. 初始化一个计数器count和当前数字now,上一个数字last
2. 实现__next__方法,每次调用返回当前数字now,并更新now为上一个数字last与当前数字now之和,同时更新last为原来的now。
3. 实现__iter__方法,返回自身作为迭代器。
4. 提供一个GetFib函数,用于生成FibIterator对象。
该迭代器可以通过以下方式使用:
```
fib = GetFib(10)
for num in fib:
print(num)
```
该代码将生成前10个斐波那契数列并输出。
import jieba from collections import Counter def read_dataset(path): labels = [] inputs = [] with open(path, 'r', encoding='utf-8') as file: for i, line in enumerate(file): line = line.strip() sample = line.split('\t') inputs.append(sample[0]) labels.append(sample[1]) return inputs, labels class MyDataset(): def init(self) -> None: self.vocab = {} self.stop_words = [] def set_stopword(self, path='data/scu_stopwords'): with open(path, 'r', encoding='utf-8') as fr: self.stop_words = [line.strip() for line in fr.readline()] def build_vocab(self, inputs, max_size='5000', min_freg=1): cnt = {} # 临时词典存储词频 for data in inputs: data = jieba.lcut(data) for word in data: if word not in cnt: cnt[word] = 1 else: cnt[word] += 1 cnt = sorted([_ for _ in cnt.items() if _[1]>=min_freg and _[0] not in self.stop_words], key=lambda t:t[1], reverse=True) self.vocab['<pad>'] = 0 if len(cnt) > max_size: i = 1 for w, _ in cnt: if len(self.vocab)>max_size: break self.vocab[w] = i i += 1 else: i = 1 for w, _ in cnt: self.vocab[w] = i i += 1 def transform(self, inputs, flag = 0): samples = [] iter = 0 for doc in inputs: if iter % 1000 == 0: print('-------%d------' % iter) doc = jieba.cut(doc) if flag==0: wordset = set(doc) # print(wordset) sample = [] for word in self.vocab.keys(): if word in wordset: sample.append(1) else: sample.append(0) elif flag == 1: sample = [0 for i in range(len(self.vocab.items()))] word_count = Counter(doc) for word in word_count.items(): if word[0] in self.vocab.keys(): id = self.vocab[word[0]] sample[id] = word[1] iter +=1 samples.append(sample) return samples def buid_tfidf_vocab(self, inputs, max_size): pass试着调参重构,提升精确率
很抱歉,您的代码片段缺少模型和评估指标等关键信息,我无法直接提供调参建议。不过,一些常见的提升精确率的方法包括:
- 调整模型结构,可以尝试更深的网络层、增加隐藏单元、添加正则化等等;
- 调整超参数,如学习率、批量大小、迭代次数等;
- 数据增强,可以尝试增加数据量、添加噪声、旋转等等;
- 特征工程,例如添加关键词、文本长度、情感分析等特征;
- 集成方法,例如结合多个模型,使用投票或加权平均等方式提升精度。
当然,具体方法需要结合您的具体任务和数据集情况进行调参和优化。
相关推荐
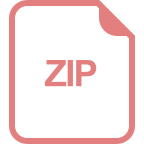
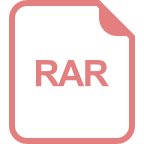












