c++利用链栈判断一个序列是否为回文序列
时间: 2023-05-20 18:02:50 浏览: 120
可以使用链栈来判断一个序列是否为回文序列。具体实现方法是将序列的前一半元素依次入栈,然后再依次出栈与后一半元素比较,如果全部相等,则该序列为回文序列。以下是 C 语言的代码实现:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_SIZE 100
typedef struct Node {
char data;
struct Node *next;
} Node, *LinkStack;
LinkStack InitStack() {
LinkStack S = (LinkStack)malloc(sizeof(Node));
S->next = NULL;
return S;
}
int IsEmpty(LinkStack S) {
return S->next == NULL;
}
void Push(LinkStack S, char x) {
Node *p = (Node*)malloc(sizeof(Node));
p->data = x;
p->next = S->next;
S->next = p;
}
char Pop(LinkStack S) {
if (IsEmpty(S)) {
printf("Stack is empty!\n");
exit(1);
}
Node *p = S->next;
char x = p->data;
S->next = p->next;
free(p);
return x;
}
int IsPalindrome(char *str) {
int len = strlen(str);
LinkStack S = InitStack();
int i;
for (i = 0; i < len / 2; i++) {
Push(S, str[i]);
}
if (len % 2 == 1) {
i++;
}
while (str[i] != '\0') {
if (Pop(S) != str[i]) {
return 0;
}
i++;
}
return 1;
}
int main() {
char str[MAX_SIZE];
printf("Please input a string: ");
scanf("%s", str);
if (IsPalindrome(str)) {
printf("The string is palindrome.\n");
} else {
printf("The string is not palindrome.\n");
}
return 0;
}
```
该程序利用链栈来实现了判断一个字符串是否为回文字符串的功能。
阅读全文
相关推荐











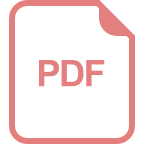