SemiCircleProgressBar 实现并支持渐变
时间: 2023-06-24 14:05:32 浏览: 41
实现一个支持渐变的半圆形进度条可以使用自定义 View 的方式,主要思路如下:
1. 继承 View,重写 onDraw 方法,在方法中绘制半圆形和进度条。
2. 在 onDraw 方法中使用 Paint 绘制半圆形和进度条。绘制半圆形可以使用 drawArc 方法,绘制渐变进度条可以使用 Shader。
3. 创建一个 SweepGradient 对象,设置渐变的颜色和位置。
4. 将 SweepGradient 对象设置给 Paint 对象的 Shader 属性,使得进度条使用渐变颜色绘制。
下面是一个简单的实现示例:
```java
public class SemiCircleProgressBar extends View {
private static final int DEFAULT_START_ANGLE = 180;
private static final int DEFAULT_SWEEP_ANGLE = 180;
private Paint mBackgroundPaint;
private Paint mProgressPaint;
private int mStartAngle = DEFAULT_START_ANGLE;
private int mSweepAngle = DEFAULT_SWEEP_ANGLE;
private int mProgressColor = Color.RED;
private int mBackgroundColor = Color.GRAY;
public SemiCircleProgressBar(Context context) {
super(context);
init();
}
public SemiCircleProgressBar(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
public SemiCircleProgressBar(Context context, AttributeSet attrs, int defStyleAttr) {
super(context, attrs, defStyleAttr);
init();
}
private void init() {
mBackgroundPaint = new Paint();
mBackgroundPaint.setAntiAlias(true);
mBackgroundPaint.setColor(mBackgroundColor);
mBackgroundPaint.setStyle(Paint.Style.STROKE);
mProgressPaint = new Paint();
mProgressPaint.setAntiAlias(true);
mProgressPaint.setStyle(Paint.Style.STROKE);
mProgressPaint.setStrokeCap(Paint.Cap.ROUND);
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int width = getWidth();
int height = getHeight();
int strokeWidth = Math.min(width, height) / 10;
mBackgroundPaint.setStrokeWidth(strokeWidth);
mProgressPaint.setStrokeWidth(strokeWidth);
RectF rectF = new RectF(strokeWidth / 2, strokeWidth / 2,
width - strokeWidth / 2, height - strokeWidth / 2);
// 绘制半圆形背景
canvas.drawArc(rectF, mStartAngle, mSweepAngle, false, mBackgroundPaint);
// 绘制渐变进度条
int[] colors = {Color.GREEN, Color.YELLOW, Color.RED};
float[] positions = {0f, 0.5f, 1f};
SweepGradient sweepGradient = new SweepGradient(width / 2, height / 2,
colors, positions);
mProgressPaint.setShader(sweepGradient);
canvas.drawArc(rectF, mStartAngle, mSweepAngle, false, mProgressPaint);
}
public void setProgressColor(int progressColor) {
mProgressColor = progressColor;
mProgressPaint.setColor(mProgressColor);
invalidate();
}
public void setBackgroundColor(int backgroundColor) {
mBackgroundColor = backgroundColor;
mBackgroundPaint.setColor(mBackgroundColor);
invalidate();
}
public void setStartAngle(int startAngle) {
mStartAngle = startAngle;
invalidate();
}
public void setSweepAngle(int sweepAngle) {
mSweepAngle = sweepAngle;
invalidate();
}
}
```
在代码中,我们使用了 SweepGradient 实现渐变,设置了颜色和位置,然后将 SweepGradient 对象设置给进度条的 Paint 对象的 Shader 属性。最终我们可以得到一个支持渐变的半圆形进度条。
相关推荐
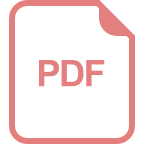














