Csharp中List<T>.Find
时间: 2024-05-26 18:16:39 浏览: 17
LastIndex 方法的作用是什么?
List<T>.FindLastIndex 方法用于搜索指定集合中符合条件的最后一个元素,并返回该元素的索引。该方法接收一个 Predicate<T> 委托作为参数,该委托用于指定要搜索的条件。如果找到符合条件的元素,则返回该元素在集合中的索引;否则返回 -1。
相关问题
public class DbContextHelper<T> where T : DbContext, new() { private static T _instance; public static T Instance { get { return _instance ?? (_instance = new T()); } } public static void Add<TEntity>(TEntity entity) where TEntity : class { using(var context = _instance) { context.Set<TEntity>().Add(entity); context.SaveChanges(); } } public static void Update<TEntity>(TEntity entity) where TEntity : class { using(var context = _instance) { context.Entry(entity).State = EntityState.Modified; context.SaveChanges(); } } public static void Delete<TEntity>(TEntity entity) where TEntity: class { using(var context = _instance) { context.Set<TEntity>().Add(entity); context.SaveChanges(); } } public static List<TEntity> GetAll<TEntity>() where TEntity : class { using (var context = _instance) { return context.Set<TEntity>().ToList(); } } public static TEntity GetById<TEntity>(object id) where TEntity : class { using (var context = _instance) { return context.Set<TEntity>().Find(id); } }如何通过导航属性获取信息
可以在`DbContextHelper`类中添加一个方法,通过导航属性获取相关信息。示例代码如下:
``` csharp
public static List<TEntity> GetAllWithNavigationProperty<TEntity>(Expression<Func<TEntity, object>> navigationPropertyPath) where TEntity : class
{
using (var context = _instance)
{
return context.Set<TEntity>().Include(navigationPropertyPath).ToList();
}
}
```
在上述代码中,`Include`方法可以通过导航属性路径指定要加载的相关实体类,从而获取相关信息。例如,如果想同时获取所有客户及其对应的订单信息,可以这样调用:
``` csharp
var customers = DbContextHelper<Customer>.GetAllWithNavigationProperty(c => c.Orders);
```
这样就可以获取所有客户及其对应的订单信息。如果需要获取特定客户及其对应的订单信息,可以使用`Where`方法来过滤数据,例如:
``` csharp
var customer = DbContextHelper<Customer>.GetAllWithNavigationProperty(c => c.Orders).FirstOrDefault(c => c.Id == 1);
```
这样就可以获取`Id`为1的客户及其对应的订单信息。
List<int> a = new List<int> { 1, 2, 3, 4 }; int b = a.Find(c => c == 2); debug.log(b)
在你的代码中,有一个小写的错误。正确的方法名是 `Debug.Log()` 而不是 `debug.log()`。
另外,`List<T>.Find()` 方法会返回满足条件的第一个元素,如果没有满足条件的元素,则返回类型的默认值。在你的代码中,因为找到了值为 2 的元素,所以 `b` 的值为 2。
所以,正确的代码应该是:
```csharp
List<int> a = new List<int> { 1, 2, 3, 4 };
int b = a.Find(c => c == 2);
Debug.Log(b);
```
这将输出 `2`。
相关推荐
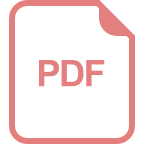
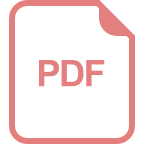
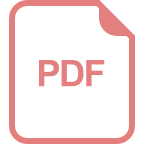












