using System.Collections.Generic; using UnityEngine; using UnityEngine.UI; using UnityEngine.EventSystems; public class Beibao : MonoBehaviour { public GameObject inventoryUI; public GameObject itemSlotPrefab; public Transform itemSlotContainer; public List<Item> items = new List<Item>(); public Dictionary<string, int> itemCounts = new Dictionary<string, int>(); private bool isInventoryOpen = false; [CreateAssetMenu(fileName = "New Item", menuName = "Inventory/Item")] public class Item : ScriptableObject { public new string name; public string description; public Sprite icon; } private void Start() { inventoryUI.SetActive(false); } private void Update() { if (Input.GetKeyDown(KeyCode.I)) { ToggleInventory(); } } public void AddItem(Item item) { items.Add(item); if (itemCounts.ContainsKey(item.name)) { itemCounts[item.name]++; } else { itemCounts[item.name] = 1; CreateItemSlot(item); } UpdateItemSlot(item); } public void RemoveItem(Item item) { items.Remove(item); if (itemCounts.ContainsKey(item.name)) { itemCounts[item.name]--; if (itemCounts[item.name] == 0) { itemCounts.Remove(item.name); DestroyItemSlot(item); } } UpdateItemSlot(item); } public void UpdateItemCount(Item item) { if (itemCounts.ContainsKey(item.name)) { itemCounts[item.name]--; UpdateItemSlot(item); } } public void ToggleInventory() { isInventoryOpen = !isInventoryOpen; inventoryUI.SetActive(isInventoryOpen); } private void CreateItemSlot(Item item) { GameObject itemSlot = Instantiate(itemSlotPrefab, itemSlotContainer); itemSlot.name = item.name; itemSlot.GetComponent<Image>().sprite = item.icon; } private void DestroyItemSlot(Item item) { Transform itemSlot = itemSlotContainer.Find(item.name); Destroy(itemSlot.gameObject); } private void UpdateItemSlot(Item item) { Transform itemSlot = itemSlotContainer.Find(item.name); Text itemText = itemSlot.GetComponentInChildren<Text>(); itemText.text = itemCounts[item.name].ToString(); } }能帮我注释一下意思吗
时间: 2024-02-10 20:15:03 浏览: 33
好的,以下是对代码的注释:
```csharp
using System.Collections.Generic; // 引入系统集合命名空间
using UnityEngine; // 引入Unity引擎命名空间
using UnityEngine.UI; // 引入Unity UI命名空间
using UnityEngine.EventSystems; // 引入Unity事件系统命名空间
public class Beibao : MonoBehaviour // 定义一个名为Beibao的MonoBehaviour类
{
public GameObject inventoryUI; // 背包UI游戏对象
public GameObject itemSlotPrefab; // 物品槽预制体游戏对象
public Transform itemSlotContainer; // 物品槽容器Transform组件
public List<Item> items = new List<Item>(); // 物品列表
public Dictionary<string, int> itemCounts = new Dictionary<string, int>(); // 物品计数字典
private bool isInventoryOpen = false; // 背包是否打开的标志
[CreateAssetMenu(fileName = "New Item", menuName = "Inventory/Item")] // 创建物品脚本对象的菜单项
public class Item : ScriptableObject // 定义一个名为Item的ScriptableObject脚本类
{
public new string name; // 物品名称
public string description; // 物品描述
public Sprite icon; // 物品图标
}
private void Start() // MonoBheaviour的Start方法
{
inventoryUI.SetActive(false); // 关闭背包UI
}
private void Update() // MonoBehaviour的Update方法
{
if (Input.GetKeyDown(KeyCode.I)) // 如果按下I键
{
ToggleInventory(); // 切换背包UI的显示状态
}
}
public void AddItem(Item item) // 添加物品到背包的方法
{
items.Add(item); // 将物品添加到物品列表中
if (itemCounts.ContainsKey(item.name)) // 如果该物品已经存在于物品计数字典中
{
itemCounts[item.name]++; // 物品计数字典中该物品的数量加1
}
else // 如果该物品不存在于物品计数字典中
{
itemCounts[item.name] = 1; // 物品计数字典中该物品的数量设置为1
CreateItemSlot(item); // 创建该物品的物品槽
}
UpdateItemSlot(item); // 更新该物品的物品槽
}
public void RemoveItem(Item item) // 从背包中移除物品的方法
{
items.Remove(item); // 将物品从物品列表中移除
if (itemCounts.ContainsKey(item.name)) // 如果该物品存在于物品计数字典中
{
itemCounts[item.name]--; // 物品计数字典中该物品的数量减1
if (itemCounts[item.name] == 0) // 如果物品计数字典中该物品的数量为0
{
itemCounts.Remove(item.name); // 将该物品从物品计数字典中移除
DestroyItemSlot(item); // 销毁该物品的物品槽
}
}
UpdateItemSlot(item); // 更新该物品的物品槽
}
public void UpdateItemCount(Item item) // 更新物品数量的方法
{
if (itemCounts.ContainsKey(item.name)) // 如果该物品存在于物品计数字典中
{
itemCounts[item.name]--; // 物品计数字典中该物品的数量减1
UpdateItemSlot(item); // 更新该物品的物品槽
}
}
public void ToggleInventory() // 切换背包UI的显示状态的方法
{
isInventoryOpen = !isInventoryOpen; // 切换背包是否打开的标志
inventoryUI.SetActive(isInventoryOpen); // 设置背包UI的显示状态
}
private void CreateItemSlot(Item item) // 创建物品槽的方法
{
GameObject itemSlot = Instantiate(itemSlotPrefab, itemSlotContainer); // 克隆物品槽预制体,并将其设置为物品槽容器的子物体
itemSlot.name = item.name; // 设置物品槽游戏对象的名称为该物品的名称
itemSlot.GetComponent<Image>().sprite = item.icon; // 设置物品槽游戏对象的图像为该物品的图标
}
private void DestroyItemSlot(Item item) // 销毁物品槽的方法
{
Transform itemSlot = itemSlotContainer.Find(item.name); // 查找物品槽容器下名为该物品名称的子物体
Destroy(itemSlot.gameObject); // 销毁该物品的物品槽游戏对象
}
private void UpdateItemSlot(Item item) // 更新物品槽的方法
{
Transform itemSlot = itemSlotContainer.Find(item.name); // 查找物品槽容器下名为该物品名称的子物体
Text itemText = itemSlot.GetComponentInChildren<Text>(); // 获取该物品的物品槽中的文本组件
itemText.text = itemCounts[item.name].ToString(); // 更新该物品的物品槽中的数量文本
}
}
```
这个代码实现了一个简单的背包系统,其中包括添加、删除、更新物品,以及显示背包UI等功能。其中 `Item` 类是一个 ScriptableObject 类,用于存储物品的名称、描述和图标等信息。 `Beibao` 类是一个 MonoBehaviour 类,用于实现背包系统的功能代码。通过将该脚本添加到游戏对象上,可以实现背包系统的功能。
相关推荐
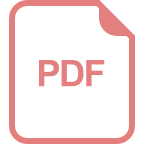
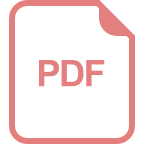














