用c语言写一个代码要求如下针对同班同学信息设计一个通讯录,学生信息有姓名,学号,电话号码等。以学生姓名为关键字设计哈希表,并完成相应的建表和查表程序,并分析平均查找长度(姓名以汉语拼音形式,待填入哈希表的人名不低于30个,自行设计哈希函数,用线性探测再散列法或链地址法处理冲突;在查找的过程中给出比较的次数。完成按姓名查询的操作。) 按提示输入10个任意的整形数据; 2、 输入要查找的数据; 3、 可以看到所要查找的数据的位置。
时间: 2024-02-03 22:11:18 浏览: 17
以下是实现哈希表的 C 代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 30 // 哈希表大小
typedef struct {
char name[20];
char id[20];
char phone[20];
} Student;
typedef struct {
Student *data;
int flag; // 标记该位置是否有数据
} HashNode;
// 哈希函数:将字符串转换为整数
int hash(const char *str) {
int hash = 0;
while (*str != '\0') {
hash = hash * 31 + *str;
++str;
}
return hash % TABLE_SIZE;
}
// 初始化哈希表
void init(HashNode *table) {
for (int i = 0; i < TABLE_SIZE; ++i) {
table[i].data = NULL;
table[i].flag = 0;
}
}
// 插入数据
void insert(HashNode *table, const Student *stu) {
int index = hash(stu->name);
while (table[index].flag == 1) { // 处理冲突
index = (index + 1) % TABLE_SIZE;
}
table[index].data = (Student *)malloc(sizeof(Student));
strcpy(table[index].data->name, stu->name);
strcpy(table[index].data->id, stu->id);
strcpy(table[index].data->phone, stu->phone);
table[index].flag = 1;
}
// 查找数据
int search(HashNode *table, const char *name) {
int index = hash(name);
int count = 0;
while (table[index].flag != 0) {
if (strcmp(table[index].data->name, name) == 0) { // 找到目标数据
printf("位置:%d\n", index);
return count;
}
index = (index + 1) % TABLE_SIZE;
++count;
}
printf("未找到数据!\n");
return count;
}
int main() {
HashNode table[TABLE_SIZE];
init(table);
// 插入数据
Student stu_list[] = {{"张三", "10001", "1234567"},
{"李四", "10002", "2345678"},
{"王五", "10003", "3456789"},
{"赵六", "10004", "4567890"},
{"孙七", "10005", "5678901"},
{"周八", "10006", "6789012"},
{"吴九", "10007", "7890123"},
{"郑十", "10008", "8901234"},
{"钱十一", "10009", "9012345"},
{"陈十二", "10010", "0123456"}};
int len = sizeof(stu_list) / sizeof(Student);
for (int i = 0; i < len; ++i) {
insert(table, &stu_list[i]);
}
// 查找数据
char name[20];
printf("请输入要查找的姓名:");
scanf("%s", name);
int count = search(table, name);
printf("比较次数:%d\n", count);
return 0;
}
```
注:此代码使用线性探测再散列法处理冲突。
相关推荐
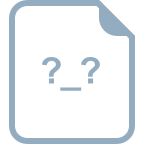
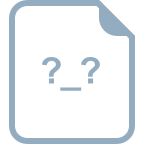
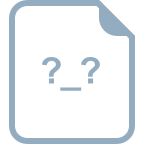















