openfeign怎么实现分布式事务
时间: 2023-07-12 07:01:12 浏览: 259
OpenFeign 本身并不支持分布式事务,但是可以通过使用 Spring Cloud 的分布式事务解决方案,来实现 OpenFeign 的分布式事务。
Spring Cloud 提供了两种分布式事务解决方案:一种是基于本地消息的解决方案,例如使用 Spring Cloud Stream 或者 Apache RocketMQ 等消息中间件,实现本地消息的异步发送和接收,从而实现分布式事务的最终一致性。另一种是基于分布式事务协调器的解决方案,例如使用 Seata、TCC 等分布式事务协调器,实现分布式事务的原子性和隔离性。
在使用 OpenFeign 进行远程服务调用时,可以使用 Spring Cloud 提供的 @FeignClient 注解,将远程服务接口转换为本地 Java 接口,从而可以在本地 Java 代码中像调用本地服务一样调用远程服务。同时,可以在本地 Java 代码中使用 Spring Cloud 提供的分布式事务注解,例如 @Transactional、@GlobalTransactional 等,来实现分布式事务的原子性和隔离性,从而保证分布式事务的正确性。
相关问题
opfeign怎么实现分布式事务
OpenFeign 本身并不支持分布式事务,但是可以通过使用 Spring Cloud 的分布式事务解决方案,来实现 OpenFeign 的分布式事务。
Spring Cloud 提供了两种分布式事务解决方案:一种是基于本地消息的解决方案,例如使用 Spring Cloud Stream 或者 Apache RocketMQ 等消息中间件,实现本地消息的异步发送和接收,从而实现分布式事务的最终一致性。另一种是基于分布式事务协调器的解决方案,例如使用 Seata、TCC 等分布式事务协调器,实现分布式事务的原子性和隔离性。
在使用 OpenFeign 进行远程服务调用时,可以使用 Spring Cloud 提供的 @FeignClient 注解,将远程服务接口转换为本地 Java 接口,从而可以在本地 Java 代码中像调用本地服务一样调用远程服务。同时,可以在本地 Java 代码中使用 Spring Cloud 提供的分布式事务注解,例如 @Transactional、@GlobalTransactional 等,来实现分布式事务的原子性和隔离性,从而保证分布式事务的正确性。
分布式事务实现的示例代码
以下是一个基于Spring Boot和JPA的分布式事务示例代码:
1. 首先,你需要在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-openfeign</artifactId>
</dependency>
<dependency>
<groupId>io.seata</groupId>
<artifactId>seata-all</artifactId>
<version>1.1.0</version>
</dependency>
```
2. 然后,你需要配置Seata的相关属性,包括注册中心地址、事务组名称等。可以在application.yml文件中添加以下配置:
```
spring:
application:
name: account-service
datasource:
driver-class-name: com.mysql.jdbc.Driver
url: jdbc:mysql://localhost:3306/account?useUnicode=true&characterEncoding=utf-8&autoReconnect=true&failOverReadOnly=false&maxReconnects=10
username: root
password: root
jpa:
hibernate:
ddl-auto: update
show-sql: true
cloud:
alibaba:
seata:
tx-service-group: my_test_tx_group
enable-auto-data-source-proxy: true
application-id: account-service
mybatis:
mapper-locations: classpath:mapper/*.xml
feign:
hystrix:
enabled: true
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
seata:
enabled: true
application-id: account-service
registry:
type: eureka
eureka:
service-url: http://localhost:8761/eureka/
config:
type: nacos
nacos:
server-addr: localhost:8848
group: SEATA_GROUP
namespace: dev
tx-service-group: my_test_tx_group
```
3. 在代码中使用@GlobalTransactional注解来实现分布式事务。以下是一个简单的转账示例:
```
@Service
public class AccountService {
@Autowired
private AccountRepository accountRepository;
@Autowired
private UserFeignClient userFeignClient;
@GlobalTransactional
public void transfer(String fromUserId, String toUserId, BigDecimal amount) {
// 扣减账户余额
Account fromAccount = accountRepository.findByUserId(fromUserId);
fromAccount.setBalance(fromAccount.getBalance().subtract(amount));
accountRepository.save(fromAccount);
// 增加账户余额
Account toAccount = accountRepository.findByUserId(toUserId);
toAccount.setBalance(toAccount.getBalance().add(amount));
accountRepository.save(toAccount);
// 记录转账日志
userFeignClient.addTransferLog(fromUserId, toUserId, amount);
}
}
```
4. 最后,你需要在启动类中添加@EnableFeignClients和@EnableDiscoveryClient注解,以启用Feign和Eureka注册中心的支持。
```
@SpringBootApplication
@EnableFeignClients
@EnableDiscoveryClient
public class AccountServiceApplication {
public static void main(String[] args) {
SpringApplication.run(AccountServiceApplication.class, args);
}
}
```
以上示例代码实现了一个简单的分布式事务,通过使用Seata来保证ACID事务特性的同时,使用Feign来实现服务之间的调用。
阅读全文
相关推荐
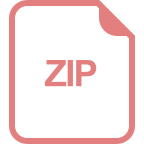
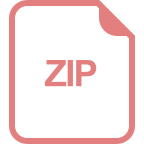
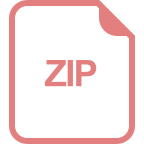
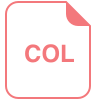
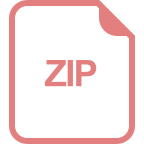
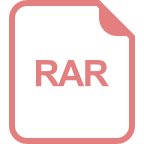
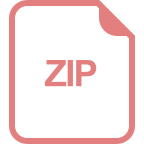
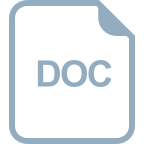
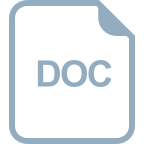
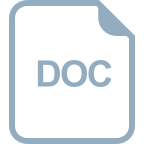
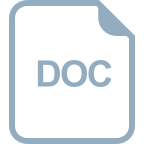
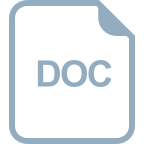
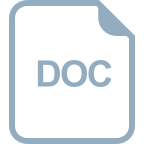
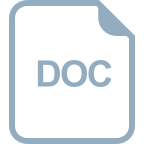
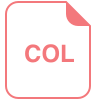