请给出b+树的java代码实现
时间: 2024-04-29 21:23:25 浏览: 62
以下是一个简单的B树Java代码实现:
```
class BTreeNode {
int[] keys; // 存储键值
int t; // 最小度数
BTreeNode[] children; // 子节点
int n; // 当前节点中的键值数量
boolean leaf; // 是否为叶子节点
public BTreeNode(int t, boolean leaf) {
this.t = t;
this.leaf = leaf;
this.keys = new int[2 * t - 1];
this.children = new BTreeNode[2 * t];
this.n = 0;
}
public void traverse() {
int i;
for (i = 0; i < this.n; i++) {
if (!this.leaf) {
this.children[i].traverse();
}
System.out.print(this.keys[i] + " ");
}
if (!this.leaf) {
this.children[i].traverse();
}
}
public BTreeNode search(int key) {
int i = 0;
while (i < this.n && key > this.keys[i]) {
i++;
}
if (this.keys[i] == key) {
return this;
}
if (this.leaf) {
return null;
}
return this.children[i].search(key);
}
public void insertNonFull(int key) {
int i = this.n - 1;
if (this.leaf) {
while (i >= 0 && this.keys[i] > key) {
this.keys[i + 1] = this.keys[i];
i--;
}
this.keys[i + 1] = key;
this.n++;
} else {
while (i >= 0 && this.keys[i] > key) {
i--;
}
if (this.children[i + 1].n == 2 * this.t - 1) {
splitChild(i + 1, this.children[i + 1]);
if (this.keys[i + 1] < key) {
i++;
}
}
this.children[i + 1].insertNonFull(key);
}
}
public void splitChild(int i, BTreeNode y) {
BTreeNode z = new BTreeNode(y.t, y.leaf);
z.n = this.t - 1;
for (int j = 0; j < this.t - 1; j++) {
z.keys[j] = y.keys[j + this.t];
}
if (!y.leaf) {
for (int j = 0; j < this.t; j++) {
z.children[j] = y.children[j + this.t];
}
}
y.n = this.t - 1;
for (int j = this.n; j >= i + 1; j--) {
this.children[j + 1] = this.children[j];
}
this.children[i + 1] = z;
for (int j = this.n - 1; j >= i; j--) {
this.keys[j + 1] = this.keys[j];
}
this.keys[i] = y.keys[this.t - 1];
this.n++;
}
}
class BTree {
BTreeNode root;
int t;
public BTree(int t) {
this.t = t;
this.root = null;
}
public void traverse() {
if (this.root != null) {
this.root.traverse();
}
}
public BTreeNode search(int key) {
if (this.root == null) {
return null;
}
return this.root.search(key);
}
public void insert(int key) {
if (this.root == null) {
this.root = new BTreeNode(this.t, true);
this.root.keys[0] = key;
this.root.n = 1;
} else {
if (this.root.n == 2 * this.t - 1) {
BTreeNode s = new BTreeNode(this.t, false);
s.children[0] = this.root;
s.splitChild(0, this.root);
int i = 0;
if (s.keys[0] < key) {
i++;
}
s.children[i].insertNonFull(key);
this.root = s;
} else {
this.root.insertNonFull(key);
}
}
}
}
```
此代码实现了B树的基本操作,包括遍历、搜索和插入。
阅读全文
相关推荐
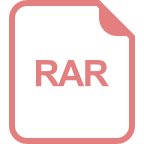














