用python写出二分类的神经网络代码
时间: 2023-09-08 19:05:29 浏览: 133
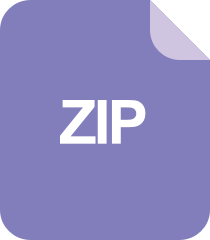
神经网络分类模型Python代码
### 回答1:
你好,我是 C 知道。以下是二分类神经网络的 Python 代码示例:
```
import numpy as np
# 定义 sigmoid 函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义神经网络类
class NeuralNetwork:
def __init__(self, input_size, hidden_size, output_size):
# 初始化权重和偏置
self.weights1 = np.random.randn(input_size, hidden_size)
self.bias1 = np.random.randn(hidden_size)
self.weights2 = np.random.randn(hidden_size, output_size)
self.bias2 = np.random.randn(output_size)
def forward(self, x):
# 前向传播
self.z1 = np.dot(x, self.weights1) + self.bias1
self.a1 = sigmoid(self.z1)
self.z2 = np.dot(self.a1, self.weights2) + self.bias2
self.a2 = sigmoid(self.z2)
return self.a2
def backward(self, x, y, output):
# 反向传播
self.error = output - y
self.delta2 = self.error * sigmoid(self.z2) * (1 - sigmoid(self.z2))
self.weights2 -= np.dot(self.a1.T, self.delta2)
self.bias2 -= np.sum(self.delta2, axis=)
self.delta1 = np.dot(self.delta2, self.weights2.T) * sigmoid(self.z1) * (1 - sigmoid(self.z1))
self.weights1 -= np.dot(x.T, self.delta1)
self.bias1 -= np.sum(self.delta1, axis=)
def train(self, x, y, epochs):
# 训练神经网络
for i in range(epochs):
output = self.forward(x)
self.backward(x, y, output)
def predict(self, x):
# 预测
output = self.forward(x)
return np.round(output)
# 测试代码
x = np.array([[, ], [, 1], [1, ], [1, 1]])
y = np.array([[], [1], [1], []])
nn = NeuralNetwork(2, 4, 1)
nn.train(x, y, 10000)
print(nn.predict(x))
```
希望这个代码示例能够帮助你!
### 回答2:
二分类的神经网络是一种广泛使用的机器学习模型,用于将输入数据划分为两个不同的类别。下面是使用Python编写二分类神经网络的一个例子:
首先,需要导入必要的库,如numpy和matplotlib。然后定义一个函数,该函数将用于执行前向传播和反向传播的操作。
```python
import numpy as np
import matplotlib.pyplot as plt
# 生成输入数据
def generate_data():
np.random.seed(0)
num_samples = 100
X = np.random.randn(num_samples, 2)
Y = np.random.randint(0, 2, size=num_samples)
return X, Y
# 定义激活函数(Sigmoid)
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义模型
def model(X, Y, num_iterations=1000, learning_rate=0.01):
num_samples, input_dim = X.shape
# 初始化参数
W = np.zeros((input_dim, 1))
b = 0
# 记录损失函数值的变化
loss_history = []
# 迭代训练
for i in range(num_iterations):
A = sigmoid(np.dot(X, W) + b) # 前向传播
cost = -np.mean(Y * np.log(A) + (1 - Y) * np.log(1 - A)) # 损失函数
# 计算梯度
dW = np.dot(X.T, (A - Y)) / num_samples
db = np.mean(A - Y)
# 更新参数
W -= learning_rate * dW
b -= learning_rate * db
# 记录每次迭代的损失函数值
loss_history.append(cost)
# 绘制损失函数的变化曲线
plt.plot(loss_history)
plt.xlabel('Iterations')
plt.ylabel('Loss')
plt.show()
return W, b
# 测试模型
X, Y = generate_data()
W, b = model(X, Y)
```
以上代码中,`generate_data` 函数用于生成随机的输入数据,其中 `X` 是一个包含特征的矩阵,而 `Y` 则是对应的类别标签。
`sigmoid` 函数是一个激活函数,用于将输出限制在0到1的范围内。 `model` 函数用于执行训练过程,其中参数 `num_iterations` 是迭代的次数,`learning_rate` 是学习率。在函数体内,首先初始化权重 `W` 和偏置项 `b` ,然后进行迭代的训练过程。每次迭代中,执行前向传播,计算损失函数,并计算梯度以进行参数更新。最后,绘制损失函数的变化曲线,并返回最终的参数值 `W` 和 `b`。
### 回答3:
下面是一段使用Python编写的二分类神经网络代码示例:
```python
import numpy as np
# 定义Sigmoid激活函数
def sigmoid(x):
return 1 / (1 + np.exp(-x))
# 定义二分类神经网络类
class NeuralNetwork:
def __init__(self, input_size):
self.input_size = input_size
self.weights = np.random.rand(input_size, 1)
self.bias = np.random.rand()
def forward(self, X):
Z = np.dot(X, self.weights) + self.bias
A = sigmoid(Z)
return A
def train(self, X, y, learning_rate, num_iterations):
for i in range(num_iterations):
A = self.forward(X)
cost = -np.mean(y * np.log(A) + (1 - y) * np.log(1 - A))
dZ = A - y
dw = (1 / len(X)) * np.dot(X.T, dZ)
db = (1 / len(X)) * np.sum(dZ)
self.weights -= learning_rate * dw
self.bias -= learning_rate * db
if (i + 1) % 100 == 0:
print(f"Cost after {i+1} iterations: {cost}")
def predict(self, X):
A = self.forward(X)
predictions = np.round(A)
return predictions
# 创建一个二分类神经网络实例
input_size = 2
nn = NeuralNetwork(input_size)
# 生成样本数据
X = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
y = np.array([[0], [1], [1], [0]])
# 训练神经网络
learning_rate = 0.1
num_iterations = 1000
nn.train(X, y, learning_rate, num_iterations)
# 使用训练好的模型进行预测
test_data = np.array([[0, 0], [0, 1], [1, 0], [1, 1]])
predictions = nn.predict(test_data)
print("Predictions:", predictions)
```
这段代码首先定义了一个Sigmoid激活函数,然后定义了一个名为`NeuralNetwork`的类,该类包含了神经网络的初始化、前向传播、训练和预测方法。在训练方法中,使用梯度下降法更新权重和偏置项,以最小化损失函数。最后,使用训练好的模型对新数据进行预测,并输出预测结果。
阅读全文
相关推荐





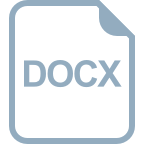
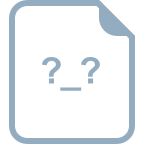
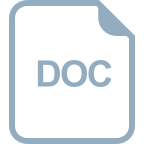
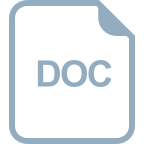
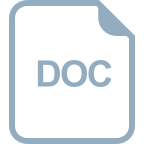
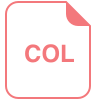
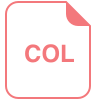



