去除表格中的数据的代码
时间: 2024-03-14 07:45:24 浏览: 117
如果你想使用编程语言来去除表格中的数据,下面是一些示例代码:
Python pandas 库:
```python
import pandas as pd
# 读取表格数据到 DataFrame
df = pd.read_excel('table.xlsx')
# 删除重复行并保存到新的 DataFrame
df_new = df.drop_duplicates()
# 将新的 DataFrame 保存到文件
df_new.to_excel('table_new.xlsx', index=False)
```
这段代码使用 pandas 库读取 Excel 文件中的表格数据,然后使用 `drop_duplicates` 方法删除重复行。最后将新的 DataFrame 保存到新的 Excel 文件中。
Java Apache POI 库:
```java
import java.io.FileInputStream;
import java.io.FileOutputStream;
import org.apache.poi.ss.usermodel.WorkbookFactory;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.Sheet;
public class RemoveDuplicates {
public static void main(String[] args) throws Exception {
// 读取 Excel 文件
FileInputStream fis = new FileInputStream("table.xlsx");
Workbook workbook = WorkbookFactory.create(fis);
fis.close();
// 获取第一个工作表
Sheet sheet = workbook.getSheetAt(0);
// 删除重复行
for (int i = 0; i <= sheet.getLastRowNum(); i++) {
for (int j = i + 1; j <= sheet.getLastRowNum(); j++) {
if (sheet.getRow(i) != null && sheet.getRow(j) != null
&& sheet.getRow(i).getCell(0) != null && sheet.getRow(j).getCell(0) != null
&& sheet.getRow(i).getCell(0).equals(sheet.getRow(j).getCell(0))) {
sheet.removeRow(sheet.getRow(j));
}
}
}
// 保存 Excel 文件
FileOutputStream fos = new FileOutputStream("table_new.xlsx");
workbook.write(fos);
fos.close();
}
}
```
这段代码使用 Apache POI 库读取 Excel 文件中的表格数据,然后遍历每一行并删除重复行。最后将修改后的数据保存回 Excel 文件。
阅读全文
相关推荐
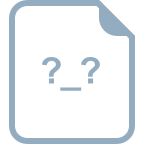

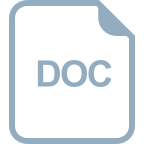
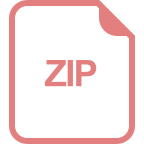
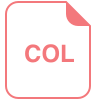











