#include<stdio.h> int isSushu(int n) { if (n < 2) return 0; for (int i = 2; i < n; i++) { if (n % i == 0) return 0; } return 1; } int * getAll(int arr[][4]) { int temp[30] = {0},tc=0; for (int i = 1; i <= 30; i++) { if(isSushu(i)) temp[tc++] = i; } int c = 0; for (int i = 0; i < tc-2; i++) { for (int j = i+1; j < tc-1; j++) { for (int k = j+1; k < tc; k++) { if (isSushu(temp[i] + temp[j] + temp[k])) { arr[c][0] = temp[i] + temp[j] + temp[k]; arr[c][1] = temp[i]; arr[c][2] = temp[j]; arr[c][3] = temp[k]; c++; } } } } int* p; return p=arr; } void dayin(int* p) { for (int i = 0; i < 50; i++) { if (*(p + 4 * i) != 0) { printf("(%d,%d,%d)=%d\n", *(p + 4 * i+1), *(p + 4 * i+2), *(p + 4 * i+3), *(p + 4 * i)); } } } int main() { int arr[50][4] = {0}; int *p=getAll(arr); dayin(p); return 0; },该代码有何问题,应该如何改正
时间: 2024-03-15 09:46:10 浏览: 26
该代码有如下问题:
1. 函数getAll返回的是一个指向局部数组的指针,在函数结束后该数组将被销毁,指针指向的内存将变成未定义行为。需要将该数组定义为静态数组或者动态分配内存。
2. 在函数getAll中,二维数组arr的下标越界,应该修改为 arr[c][0] = temp[i] + temp[j] + temp[k];。
3. 在函数dayin中,使用指针进行遍历时,指针应该指向int类型的指针,而不是void类型的指针。
以下是修改后的代码:
```
#include<stdio.h>
#include<stdlib.h>
int isSushu(int n)
{
if (n < 2)
return 0;
for (int i = 2; i < n; i++)
{
if (n % i == 0)
return 0;
}
return 1;
}
int* getAll(int arr[][4])
{
int temp[30] = {0},tc=0;
for (int i = 1; i <= 30; i++)
{
if(isSushu(i))
temp[tc++] = i;
}
int c = 0;
for (int i = 0; i < tc-2; i++)
{
for (int j = i+1; j < tc-1; j++)
{
for (int k = j+1; k < tc; k++)
{
if (isSushu(temp[i] + temp[j] + temp[k]))
{
arr[c][0] = temp[i] + temp[j] + temp[k];
arr[c][1] = temp[i];
arr[c][2] = temp[j];
arr[c][3] = temp[k];
c++;
}
}
}
}
return (int*)arr;
}
void dayin(int* p)
{
for (int i = 0; i < 50; i++)
{
if (*(p + 4 * i) != 0)
{
printf("(%d,%d,%d)=%d\n", *(p + 4 * i+1), *(p + 4 * i+2), *(p + 4 * i+3), *(p + 4 * i));
}
}
}
int main()
{
int arr[50][4] = {0};
int* p = getAll(arr);
dayin(p);
return 0;
}
```
相关推荐
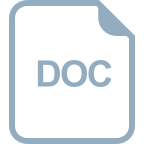
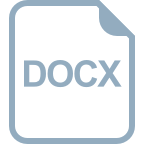
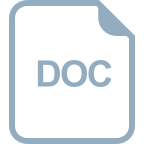
















