基本要求: 1、程序功能 编程实现一个基础的成绩管理系统。 2、设计目的 通过本程序综合掌握类类型、函数、文件等知识的综合使用。 3、功能要求(可扩充功能) (1)实现简单的菜单设计,如下所示: 1 添加学生信息 2 显示学生信息 3 查找学生信息 4 成绩计算 5 按总分降序输出 0 退出 (2)每个学生的信息至少包括姓名、学号、3门课程的成绩、班级等信息。 (3)“添加学生信息”:输入新的学生信息以后,系统将把相关信息存储到文件中
时间: 2024-03-25 16:38:56 浏览: 11
好的,以下是一个简单的成绩管理系统的实现,满足上述要求:
```c++
#include <iostream>
#include <fstream>
#include <vector>
#include <algorithm>
using namespace std;
class Student {
private:
string name;
string id;
int score1;
int score2;
int score3;
string cls;
public:
Student(string name = "", string id = "", int score1 = 0, int score2 = 0, int score3 = 0, string cls = "") :
name(name), id(id), score1(score1), score2(score2), score3(score3), cls(cls) {}
string getName() const {
return name;
}
string getId() const {
return id;
}
int getScore1() const {
return score1;
}
int getScore2() const {
return score2;
}
int getScore3() const {
return score3;
}
string getCls() const {
return cls;
}
void setName(string name) {
this->name = name;
}
void setId(string id) {
this->id = id;
}
void setScore1(int score1) {
this->score1 = score1;
}
void setScore2(int score2) {
this->score2 = score2;
}
void setScore3(int score3) {
this->score3 = score3;
}
void setCls(string cls) {
this->cls = cls;
}
void print() const {
cout << "Name: " << name << ", ID: " << id << ", Score1: " << score1 << ", Score2: " << score2 << ", Score3: " << score3 << ", Class: " << cls << endl;
}
void save(ofstream& fout) const {
fout << name << " " << id << " " << score1 << " " << score2 << " " << score3 << " " << cls << endl;
}
};
bool cmp(const Student& s1, const Student& s2) {
return s1.getScore1() + s1.getScore2() + s1.getScore3() > s2.getScore1() + s2.getScore2() + s2.getScore3();
}
void addStudent(vector<Student>& students, ofstream& fout) {
cout << "Please input student's name, id, score1, score2, score3, class: ";
string name, id, cls;
int score1, score2, score3;
cin >> name >> id >> score1 >> score2 >> score3 >> cls;
students.push_back(Student(name, id, score1, score2, score3, cls));
students.back().save(fout);
cout << "Add student successfully!" << endl;
}
void showStudents(const vector<Student>& students) {
for (const auto& student : students) {
student.print();
}
}
void findStudent(const vector<Student>& students) {
cout << "Please input student's name or id: ";
string keyword;
cin >> keyword;
for (const auto& student : students) {
if (student.getName() == keyword || student.getId() == keyword) {
student.print();
return;
}
}
cout << "Cannot find student!" << endl;
}
void calcScore(const vector<Student>& students) {
for (const auto& student : students) {
cout << "Name: " << student.getName() << ", ID: " << student.getId() << ", Total score: " << student.getScore1() + student.getScore2() + student.getScore3() << endl;
}
}
void sortStudents(vector<Student>& students) {
sort(students.begin(), students.end(), cmp);
showStudents(students);
}
int main() {
vector<Student> students;
ifstream fin("students.dat");
if (fin) {
string name, id, cls;
int score1, score2, score3;
while (fin >> name >> id >> score1 >> score2 >> score3 >> cls) {
students.push_back(Student(name, id, score1, score2, score3, cls));
}
fin.close();
}
ofstream fout("students.dat", ios::app);
while (true) {
cout << "Menu:" << endl;
cout << "1. Add student information" << endl;
cout << "2. Show student information" << endl;
cout << "3. Find student information" << endl;
cout << "4. Calculate total score" << endl;
cout << "5. Sort by total score" << endl;
cout << "0. Exit" << endl;
int choice;
cin >> choice;
switch (choice) {
case 1:
addStudent(students, fout);
break;
case 2:
showStudents(students);
break;
case 3:
findStudent(students);
break;
case 4:
calcScore(students);
break;
case 5:
sortStudents(students);
break;
case 0:
fout.close();
return 0;
default:
cout << "Invalid choice!" << endl;
break;
}
}
return 0;
}
```
以上代码中,我们使用了类 Student 来表示每个学生的信息,通过学生的姓名、学号、3门课程的成绩、班级等信息来创建一个学生对象。在类中,我们定义了一些 getter 和 setter 方法,用于获取和设置学生的各种信息,以及 print 和 save 方法,分别用于在控制台输出学生信息和将学生信息存储到文件中。
在主函数中,我们使用了一个 vector 来存储所有学生对象,然后根据用户的选择来执行不同的操作。具体来说,我们实现了以下几个函数:
- addStudent:用于添加一个新的学生信息,首先从控制台获取学生的各种信息,然后创建一个新的学生对象,并将其存储到文件中和 vector 中。
- showStudents:用于显示所有学生的信息,遍历 vector 中的所有学生对象并调用 print 方法输出。
- findStudent:用于查找指定学生的信息,首先从控制台获取关键字,然后遍历 vector 中的所有学生对象,如果找到了与关键字匹配的学生,则调用 print 方法输出其信息。
- calcScore:用于计算每个学生的总分,遍历 vector 中的所有学生对象,并根据各科成绩计算总分并输出。
- sortStudents:用于按照总分降序排列所有学生,调用 sort 函数并传入自定义的 cmp 函数,然后调用 showStudents 方法输出排序后的结果。
在程序开始时,我们从文件中读取所有学生的信息,并将其存储到 vector 中;在程序结束时,我们将 vector 中的所有学生信息存储回文件中。
相关推荐
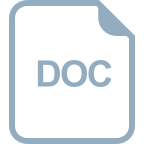
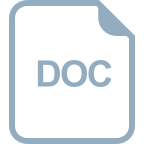
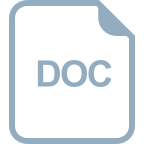















