import open3d as o3d import numpy as np pcd = o3d.io.read_point_cloud("data.pcd") points = np.asarray(pcd.points) bbox = pcd.get_axis_aligned_bounding_box() bbox_points = np.asarray(bbox.get_box_points()) vis = o3d.visualization.Visualizer() vis.create_window() vis.add_geometry(pcd) bbox_lines = [[0,1],[1,2],[2,3],[3,0], [4,5],[5,6],[6,7],[7,4], [0,4],[1,5],[2,6],[3,7]] bbox_colors = [[1,0,0] for i in range(len(bbox_lines))] line_set = o3d.geometry.LineSet( points=o3d.utility.Vector3dVector(bbox_points), lines=o3d.utility.Vector2iVector(bbox_lines), ) line_set.colors = o3d.utility.Vector3dVector(bbox_colors) vis.add_geometry(line_set) labels = np.zeros(points.shape[0]) eps = 0.1 min_points = 10 for i in range(points.shape[0]): if labels[i] != 0: continue neighbors = np.where(np.sum((points - points[i])2, axis=1) < eps2)[0] if neighbors.shape[0] < min_points: labels[i] = -1 else: labels[neighbors] = i+1 cluster_colors = [[np.random.uniform(0, 1), np.random.uniform(0, 1), np.random.uniform(0, 1)] for i in range(np.max(labels))] for i in range(np.max(labels)): if i == -1: continue cluster_points = points[labels==i,:] cluster_pcd = o3d.geometry.PointCloud() cluster_pcd.points = o3d.utility.Vector3dVector(cluster_points) cluster_pcd.paint_uniform_color(cluster_colors[i]) vis.add_geometry(cluster_pcd) vis.run() vis.destroy_window() open3d未响应
时间: 2023-06-19 08:03:43 浏览: 68
这段代码使用了Open3D库来读取点云数据,并进行基于DBSCAN算法的聚类,并且还使用了Open3D的可视化功能来显示点云和聚类结果。但是,你提供的代码有一些语法错误,可能导致Open3D无法运行。你可以尝试以下修改:
1. 在第9行之前添加 `import open3d.visualization`,以便使用Open3D的可视化功能。
2. 在第22行之前添加 `import random`,以便使用 `np.random.uniform()` 函数。
3. 在第14行中,将 `(points - points[i])2` 修改为 `(points - points[i])**2`,以便进行平方运算。
在修改完成后,你可以尝试重新运行代码,并检查是否能够正常运行。如果仍然有问题,你可以提供更详细的错误信息或代码上下文,以便更好地帮助你解决问题。
相关问题
import os import open3d as o3d import numpy as np import copy path="D:/data/Dynamo_Code/Revit_Model/Revit_Model/" #待读取的文件夹 path_list=os.listdir(path) for filename in path_list: importpath_name = os.path.join(path,filename) mesh = o3d.io.read_triangle_mesh(importpath_name) point_cloud = mesh.sample_points_uniformly(number_of_points=1000) outpath = "C:/Users/Hupan/Desktop/revit_model/revit_model/" outpath_name = os.path.join(outpath,filename) o3d.io.write_point_cloud(outpath_name , point_cloud) 以上代码是通过open3d库,批量将stl格式文件转化为pcd格式文件
你的代码看起来已经实现了使用Open3D库将STL格式文件转换为PCD格式文件的功能,只是需要一些修改来确保它可以正常工作。下面是修改后的代码:
```python
import os
import open3d as o3d
path = "D:/data/Dynamo_Code/Revit_Model/Revit_Model/" # 待读取的文件夹
path_list = os.listdir(path)
outpath = "C:/Users/Hupan/Desktop/revit_model/revit_model/"
for filename in path_list:
importpath_name = os.path.join(path, filename)
mesh = o3d.io.read_triangle_mesh(importpath_name)
point_cloud = mesh.sample_points_uniformly(number_of_points=1000)
file_name, file_extension = os.path.splitext(filename)
outpath_name = os.path.join(outpath, file_name + ".pcd")
o3d.io.write_point_cloud(outpath_name, point_cloud)
```
主要修改如下:
1. 将导入路径和导出路径的定义放在循环外部,避免每次循环都重新定义路径。
2. 使用 `os.path.splitext()` 函数来获取文件名和文件扩展名,以便在导出时将扩展名更改为 `.pcd`。
3. 修改导出路径的拼接方式,将新的文件名与导出路径合并。
请确保你已经正确安装了Open3D库,并且将路径替换为你自己的文件夹路径。这样,代码就能够按照你的需求将STL文件转换为PCD文件。
import numpy as np import open3d as o3d # 读取pcd文件 pcd = o3d.io.read_point_cloud(r"E:\BISHE\pcd\output2.pcd") # 获取点云数据 points = np.asarray(pcd.points) # 计算包围盒体积 bbox_volume = pcd.get_axis_aligned_bounding_box().volume() # 计算点云体积 point_volume = points.shape[0] * np.mean(np.linalg.norm(points, axis=1)) ** 3 / 6 * np.pi # 打印结果 print("Bounding box volume:", bbox_volume) print("Point cloud volume:", point_volume)请在这段函数最后添加一些代码,功能为生成一个txt文件,内容为bbox_volume和point_volume这两个变量
可以在最后添加如下代码来生成一个txt文件:
```
# 生成txt文件
with open('volume.txt', 'w') as f:
f.write("Bounding box volume: {}\n".format(bbox_volume))
f.write("Point cloud volume: {}\n".format(point_volume))
print("Volume data saved to volume.txt")
```
这段代码会在程序执行完毕后,生成一个名为 "volume.txt" 的文件,并将 bbox_volume 和 point_volume 的值写入文件中。
相关推荐
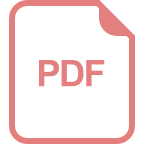
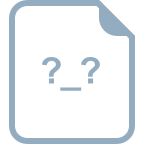
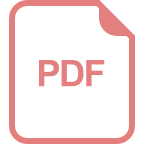












