C语言实现tar文件的打包
时间: 2023-09-17 21:15:00 浏览: 242
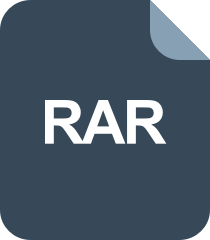
纯asp实现tar格式文件打包及解包

在C语言中,可以使用libtar库来实现tar文件的打包。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include <utime.h>
#include <tar.h>
int create_tar(const char *filename, const char *files[], int count) {
int fd;
size_t i, bytes_written;
struct stat stat_buf;
struct utimbuf time_buf;
char header_buf[T_BLOCKSIZE];
tar_header_t *header = (tar_header_t *) header_buf;
memset(header_buf, 0, T_BLOCKSIZE);
header->typeflag = REGTYPE;
for (i = 0; i < count; ++i) {
if (stat(files[i], &stat_buf) != 0) {
perror("stat");
return -1;
}
strncpy(header->name, files[i], T_NAMESIZE);
snprintf(header->size, T_SIZESIZE, "%011lo", (unsigned long) stat_buf.st_size);
snprintf(header->mode, T_MODESIZE, "%07o", stat_buf.st_mode & 07777);
snprintf(header->mtime, T_MTIMELEN, "%011lo", (unsigned long) stat_buf.st_mtime);
snprintf(header->uid, T_UIDSIZE, "%07o", stat_buf.st_uid);
snprintf(header->gid, T_GIDSIZE, "%07o", stat_buf.st_gid);
snprintf(header->magic, T_MAGICSIZE, "%s", TMAGIC);
snprintf(header->version, T_VERSIONSIZE, "%s", TVERSION);
snprintf(header->uname, T_UNAMESIZE, "%s", "");
snprintf(header->gname, T_GNAMESIZE, "%s", "");
snprintf(header->devmajor, T_DEVSIZE, "%s", "");
snprintf(header->devminor, T_DEVSIZE, "%s", "");
if ((fd = open(files[i], O_RDONLY)) == -1) {
perror("open");
return -1;
}
bytes_written = write(1, header_buf, T_BLOCKSIZE);
if (bytes_written != T_BLOCKSIZE) {
perror("write");
return -1;
}
bytes_written = 0;
while (bytes_written < stat_buf.st_size) {
bytes_written += sendfile(1, fd, NULL, stat_buf.st_size);
if (bytes_written == -1) {
perror("sendfile");
return -1;
}
}
close(fd);
time_buf.actime = stat_buf.st_atime;
time_buf.modtime = stat_buf.st_mtime;
if (utime(files[i], &time_buf) != 0) {
perror("utime");
return -1;
}
if (stat(files[i], &stat_buf) != 0) {
perror("stat");
return -1;
}
if (S_ISDIR(stat_buf.st_mode)) {
memset(header_buf, 0, T_BLOCKSIZE);
header->typeflag = DIRTYPE;
snprintf(header->name, T_NAMESIZE, "%s/", files[i]);
bytes_written = write(1, header_buf, T_BLOCKSIZE);
if (bytes_written != T_BLOCKSIZE) {
perror("write");
return -1;
}
}
}
memset(header_buf, 0, T_BLOCKSIZE);
bytes_written = write(1, header_buf, T_BLOCKSIZE);
if (bytes_written != T_BLOCKSIZE) {
perror("write");
return -1;
}
return 0;
}
int main(int argc, char *argv[]) {
char *files[] = {"file1.txt", "file2.txt", "directory"};
if (create_tar("archive.tar", files, 3) != 0) {
fprintf(stderr, "Failed to create archive\n");
return 1;
}
return 0;
}
```
该代码会将给定的文件和目录打包成一个名为`archive.tar`的tar文件,并输出到标准输出。其中,`create_tar`函数接受一个文件名、一个文件名列表和文件数作为参数,返回0表示成功,-1表示失败。在函数中,首先使用`stat`函数获取每个文件的元数据信息,然后填充tar头部,写入tar文件。如果文件是一个目录,需要单独处理,并将目录名后面加上斜线。最后,需要在tar文件结尾写入一个空的tar块,以表示tar文件结束。
阅读全文
相关推荐
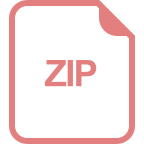
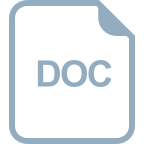

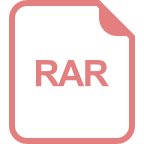
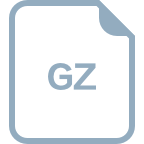
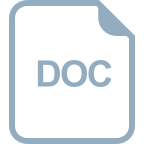
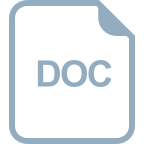
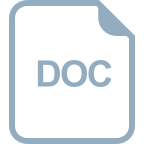
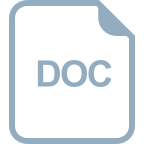
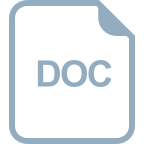
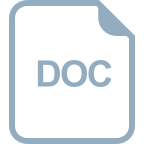
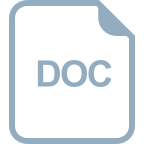
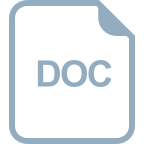
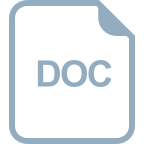
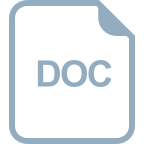
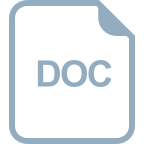
