将记录的位姿信息作为程序的输入,使T265模组在启动后直接跳转到该位姿,具体代码
时间: 2024-05-06 10:22:05 浏览: 18
由于T265模组的位姿信息是通过IMU和视觉传感器共同计算得出的,因此在启动后无法直接跳转到指定的位姿,需要先进行初始化并进行一定的运动,以便T265模组获取足够的传感器数据来计算出当前的位姿。
以下是一个简单的示例代码,可以在T265模组初始化后,将机器人移动到指定的位置和姿态,并将当前的位姿信息记录下来,然后作为程序的输入,在下次启动时直接跳转到该位姿:
```python
import pyrealsense2 as rs
import numpy as np
# 创建一个pipeline
pipeline = rs.pipeline()
# 创建config对象,用于配置T265模组
config = rs.config()
# 启用T265内置IMU
config.enable_device('T265', True)
# 启用T265的视觉传感器
config.enable_stream(rs.stream.pose)
# 开始采集
pipeline.start(config)
# 等待T265模组初始化
while True:
frames = pipeline.wait_for_frames()
pose = frames.get_pose_frame()
if pose:
pose_data = pose.get_pose_data()
if not np.isnan(pose_data.translation.x):
break
# 记录当前位姿信息
init_pose = pose_data
# 将机器人移动到指定的位置和姿态
# ...
# 关闭pipeline
pipeline.stop()
# 保存当前位姿信息
np.save('init_pose.npy', init_pose)
```
在下次启动时,可以读取之前保存的位姿信息,并将其作为程序的输入,然后设置T265模组的起始位姿为该位姿即可:
```python
import pyrealsense2 as rs
import numpy as np
# 创建一个pipeline
pipeline = rs.pipeline()
# 创建config对象,用于配置T265模组
config = rs.config()
# 启用T265内置IMU
config.enable_device('T265', True)
# 启用T265的视觉传感器
config.enable_stream(rs.stream.pose)
# 读取之前保存的位姿信息
init_pose = np.load('init_pose.npy', allow_pickle=True)
# 设置T265模组的起始位姿为init_pose
pose_sensor = pipeline.get_active_profile().get_device().first_pose_sensor()
pose_sensor.set_pose(rs.Pose(init_pose.rotation, init_pose.translation))
# 开始采集
pipeline.start(config)
# 主循环
while True:
frames = pipeline.wait_for_frames()
pose = frames.get_pose_frame()
if pose:
pose_data = pose.get_pose_data()
# 处理位姿信息
# ...
```
相关推荐
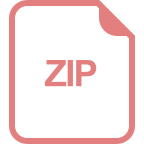














