编写一个面向对象的程序来计算矩形的面积。
时间: 2024-02-13 08:07:38 浏览: 30
好的,我可以帮你编写这个程序。
首先,我们需要定义一个矩形类,其中包含矩形的长和宽属性,以及一个计算面积的方法。下面是一个简单的 Python 代码示例:
```python
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def area(self):
return self.length * self.width
```
在这个代码中,我们定义了一个名为 `Rectangle` 的类,它有两个属性 `length` 和 `width`,分别表示矩形的长和宽。`__init__` 方法用于初始化这两个属性,接受两个参数 `length` 和 `width`。另外,我们还定义了一个名为 `area` 的方法,用于计算矩形的面积。
使用这个类,我们可以创建一个矩形对象,并调用 `area` 方法来计算它的面积。例如:
```python
rect = Rectangle(5, 3)
print(rect.area()) # 输出 15
```
在这个代码中,我们创建了一个长为 5,宽为 3 的矩形对象 `rect`,并调用它的 `area` 方法来计算它的面积,最终输出结果为 15。
希望这个程序能够满足你的需求,如果你有其他问题或需求,可以随时问我。
相关问题
编写一个计算矩形面积的面向对象程序。
好的,以下是使用C++实现的计算矩形面积的面向对象程序:
```cpp
#include <iostream>
using namespace std;
class Rectangle {
private:
float length;
float width;
public:
Rectangle(float l, float w) {
length = l;
width = w;
}
float getArea() {
return length * width;
}
};
int main() {
float l, w;
cout << "请输入矩形的长度和宽度:";
cin >> l >> w;
Rectangle r(l, w);
cout << "矩形的面积为:" << r.getArea() << endl;
return 0;
}
```
在该程序中,我们定义了一个名为`Rectangle`的类,它包含两个私有成员变量`length`和`width`,以及一个公有成员函数`getArea`,用于计算矩形的面积。
在`main`函数中,我们先从用户输入获取矩形的长度和宽度,然后创建了一个名为`r`的`Rectangle`对象,并将长度和宽度作为构造函数的参数传递进去。最后,我们调用`getArea`函数计算矩形的面积,并使用`cout`输出结果。
请注意,C++是一种支持面向对象编程的编程语言,它提供了类和对象的概念,使得我们可以更方便地进行面向对象编程。
用C++编写一个面向对象的程序来计算矩形的面积。
一个简单的面向对象的矩形类可以包含以下成员变量和成员函数:
```
class Rectangle {
private:
double width; // 矩形的宽
double height; // 矩形的高
public:
Rectangle(double w, double h); // 构造函数
double getArea(); // 计算矩形面积
};
```
其中,构造函数用于初始化矩形的宽和高,getArea() 函数用于计算矩形的面积。类的实现如下:
```
Rectangle::Rectangle(double w, double h) {
width = w;
height = h;
}
double Rectangle::getArea() {
return width * height;
}
```
然后可以在 main 函数中创建一个 Rectangle 对象,调用它的 getArea() 函数来计算矩形的面积,如下所示:
```
int main() {
Rectangle rect(3.0, 4.0); // 创建一个宽为 3,高为 4 的矩形对象
double area = rect.getArea();
cout << "The area of the rectangle is: " << area << endl;
return 0;
}
```
输出结果为:
```
The area of the rectangle is: 12
```
相关推荐
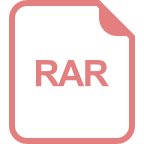
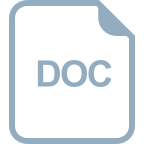
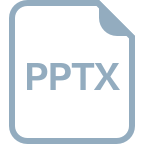












