请帮我优化代码:#include <iostream> using namespace std; int main() { bool isOut[1005] = {}; int n,m,p=0; cin>>n>>m; for(int i=1;i<=n;++i) { for(int j=0;j<m-1;++j) { while(isOut[p] == true) p = (p+1)%n; p = (p+1)%n; } while(isOut[p] == true) p = (p+1)%n; isOut[p] = true; cout<<p+1<<' '; } return 0; }
时间: 2023-03-21 14:01:19 浏览: 58
很高兴为您提供帮助!在进行代码优化之前,需要了解您的代码的目标以及您想要优化的方面。
常见的代码优化方法包括:
1. 减少代码的复杂度,如使用简洁的算法或数据结构。
2. 使用更快的数据结构或算法,例如使用哈希表代替暴力搜索。
3. 对于需要高效执行的代码块,使用优化的代码实现,如使用 C++ 等低级语言或使用编译器优化。
4. 分析代码的性能瓶颈,并使用性能分析工具优化瓶颈代码。
如果您有具体的代码,可以提供给我,我可以为您提供更具体的建议。
相关问题
栈是一种“先进后出”的数据结构,它具有如下操作: push,将元素压入栈中 pop,将栈顶元素弹出 size,返回栈中元素个数 empty,判断栈是否为空 现在要求利用标准库中的vector类实现栈,其声明如下: template<typename T> class Mystack{ private: vector<T> elems; public: void push(T&& e); void pop(T& e); int size() const; bool empty() const; }; 请实现该类,并通过相应的测试程序。 测试程序: #include<vector> #include<iostream> using namespace std; template<typename T> class Mystack{ private: vector<T> elems; public: void push(T&& e); void pop(T& e); int size() const; bool empty() const; }; /* 请在这里填写答案 */ int main() { Mystack<int> s; s.push(10); s.push(20); s.push(30); cout<<s.size()<<endl; while(!s.empty()) { int e; s.pop(e); cout<<e<<endl; } return 0; } 输入样例: 输出样例: 3 30 20 10。将类中的函数写在类外。
完整代码如下:
```cpp
#include<vector>
#include<iostream>
using namespace std;
template<typename T>
class Mystack {
private:
vector<T> elems;
public:
void push(T&& e);
void pop(T& e);
int size() const;
bool empty() const;
};
template<typename T>
void Mystack<T>::push(T&& e) {
elems.push_back(forward<T>(e));
}
template<typename T>
void Mystack<T>::pop(T& e) {
if (elems.empty()) {
throw out_of_range("stack is empty");
}
e = move(elems.back());
elems.pop_back();
}
template<typename T>
int Mystack<T>::size() const {
return elems.size();
}
template<typename T>
bool Mystack<T>::empty() const {
return elems.empty();
}
int main() {
Mystack<int> s;
s.push(10);
s.push(20);
s.push(30);
cout << s.size() << endl;
while (!s.empty()) {
int e;
s.pop(e);
cout << e << endl;
}
return 0;
}
```
注意,我们需要将函数的实现写在类外,并在函数名前面加上模板声明 `template<typename T>`。此外,在 `push` 函数中,我们使用了右值引用来避免拷贝。在 `pop` 函数中,我们使用了 `move` 来将栈顶元素移动到传入的参数中,避免了不必要的拷贝。同时,我们在 `pop` 函数中加入了越界检查,并抛出异常。
帮我改对#include<iostream> #include<fstream> #include<string> #include<vector> #include <algorithm> using namespace std; enum Grade { E=0,D,C,B,A }; class Student { public: int number; string name; double score; }; ostream& operator<<(ostream& out, Student& p) { return out; } bool compare(const Student& a, const Student& b) { return a.number < b.number; } void printVector(vector<Student>&students) { for (vector<Student>::iterator it = students.begin(); it != students.end(); it++) { cout << *it << " "; } cout << endl; } void test01() { vector<Student>students; printVector(students); } int main() { // 学生信息导入 ifstream infile; infile.open("student.txt",ios::in); if (!infile.is_open()) { cout << "文件打开失败" << endl; } vector<Student> students(27); char buf[] = { 0 }; while (infile>>buf) { cout << buf << endl; } infile.close(); // 成绩查询 int choice = 0; while (choice != 4) { cout << "请输入您要进行的操作:\n"; cout << "1. 按学号查询\n"; cout << "2. 按姓名查询\n"; cout << "3. 统计班级成绩\n"; cout << "4. 退出程序\n"; cin >> choice; if (choice == 1) { int number; cout << "请输入学号:\n"; cin >> number; auto it = find_if(students.begin(), students.end(), [number](const Student& s) { return s.number == number; }); if (it != students.end()) { cout << "学号\t姓名\t成绩\n"; cout << it->number << "\t" << it->name << "\t" << it->score << "\n"; } else { cout << "查无此人!\n"; } } else if (choice == 2) { string name; cout << "请输入姓名:\n"; cin >> name; auto it = find_if(students.begin(), students.end(), [name](const Student& s) { return s.name == name; }); if (it != students.end()) {
好的,我来帮你看一下代码,发现代码中存在一些问题,我来解释一下并给你修改建议。
1. 在函数`ostream& operator<<(ostream& out, Student& p)`中,你没有输出学生信息,需要在函数体内添加输出语句,比如:
```cpp
out << p.number << "\t" << p.name << "\t" << p.score << "\n";
```
2. 在函数`printVector(vector<Student>&students)`中,你使用了迭代器,但是你没有在`Student`类中重载`<<`运算符,所以无法输出学生信息。你需要在`Student`类中添加重载函数,比如:
```cpp
friend ostream& operator<<(ostream& out, Student& p);
```
同时也需要将`printVector`函数中的输出语句修改为:
```cpp
cout << *it << " ";
```
3. 在`main`函数中,你定义了一个`vector<Student> students(27)`,但是没有将数据读入其中,所以后续查询操作时会出错。你需要在读取文件时,将读取到的学生信息添加到`students`中,比如:
```cpp
Student stu;
int i = 0;
while (infile >> stu.number >> stu.name >> stu.score) {
students[i] = stu;
i++;
}
```
4. 在`main`函数中,你使用了`auto`关键字,但是没有包含`<iterator>`头文件,所以编译时会出错。你需要在头文件中添加`#include<iterator>`。
5. 在`main`函数中,你使用了`find_if`算法查找学生信息,但是你没有对`students`进行排序,所以查找时需要使用自定义的比较函数`compare`,你需要在查找前对`students`进行排序,比如:
```cpp
sort(students.begin(), students.end(), compare);
```
6. 在`main`函数中,你没有处理用户输入非法字符的情况,比如用户输入了一个字符,导致程序出错,你需要添加输入检测。
综上,你的代码修改后应该如下:
相关推荐
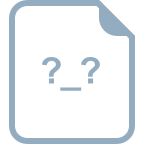










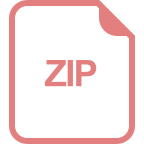
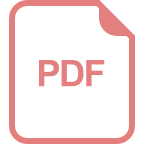
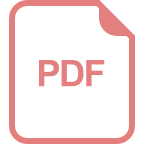