编写一个能够用鼠标操作的C语言围棋程序
时间: 2023-06-25 13:03:41 浏览: 52
编写一个能够用鼠标操作的C语言围棋程序需要使用图形界面库,比如Windows下的WinAPI或者Linux下的GTK+等。以下是一个简单的围棋程序的代码示例,使用了WinAPI:
```c
#include <windows.h>
#include <stdio.h>
#define BOARD_SIZE 19
#define BLOCK_SIZE 30
#define BOARD_OFFSET 50
#define WINDOW_WIDTH 700
#define WINDOW_HEIGHT 700
LRESULT CALLBACK WndProc(HWND, UINT, WPARAM, LPARAM);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, PSTR szCmdLine, int iCmdShow)
{
static TCHAR szAppName[] = TEXT("SimpleGo");
HWND hwnd;
MSG msg;
WNDCLASS wndclass;
wndclass.style = CS_HREDRAW | CS_VREDRAW;
wndclass.lpfnWndProc = WndProc;
wndclass.cbClsExtra = 0;
wndclass.cbWndExtra = 0;
wndclass.hInstance = hInstance;
wndclass.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wndclass.hCursor = LoadCursor(NULL, IDC_ARROW);
wndclass.hbrBackground = (HBRUSH)GetStockObject(WHITE_BRUSH);
wndclass.lpszMenuName = NULL;
wndclass.lpszClassName = szAppName;
if (!RegisterClass(&wndclass))
{
MessageBox(NULL, TEXT("This program requires Windows NT!"), szAppName, MB_ICONERROR);
return 0;
}
hwnd = CreateWindow(szAppName, TEXT("SimpleGo"), WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, WINDOW_WIDTH, WINDOW_HEIGHT,
NULL, NULL, hInstance, NULL);
ShowWindow(hwnd, iCmdShow);
UpdateWindow(hwnd);
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT message, WPARAM wParam, LPARAM lParam)
{
static int board[BOARD_SIZE][BOARD_SIZE];
static int turn = 1;
static int black_stones = 0;
static int white_stones = 0;
HDC hdc;
PAINTSTRUCT ps;
RECT rect;
switch (message)
{
case WM_CREATE:
memset(board, 0, sizeof(board));
return 0;
case WM_SIZE:
return 0;
case WM_PAINT:
hdc = BeginPaint(hwnd, &ps);
GetClientRect(hwnd, &rect);
DrawBoard(hdc, rect);
DrawStones(hdc, board);
EndPaint(hwnd, &ps);
return 0;
case WM_LBUTTONUP:
int x = LOWORD(lParam);
int y = HIWORD(lParam);
int row = (y - BOARD_OFFSET) / BLOCK_SIZE;
int col = (x - BOARD_OFFSET) / BLOCK_SIZE;
if (row < 0 || row >= BOARD_SIZE || col < 0 || col >= BOARD_SIZE)
break;
if (board[row][col] == 0)
{
board[row][col] = turn;
if (turn == 1)
black_stones++;
else
white_stones++;
turn = 3 - turn;
InvalidateRect(hwnd, NULL, TRUE);
}
return 0;
case WM_DESTROY:
PostQuitMessage(0);
return 0;
}
return DefWindowProc(hwnd, message, wParam, lParam);
}
void DrawBoard(HDC hdc, RECT rect)
{
HPEN hPen = CreatePen(PS_SOLID, 1, RGB(0, 0, 0));
HPEN hOldPen = SelectObject(hdc, hPen);
int x, y, i;
for (i = 0; i < BOARD_SIZE; i++)
{
x = BOARD_OFFSET + i * BLOCK_SIZE;
y = BOARD_OFFSET;
MoveToEx(hdc, x, y, NULL);
LineTo(hdc, x, y + (BOARD_SIZE - 1) * BLOCK_SIZE);
}
for (i = 0; i < BOARD_SIZE; i++)
{
x = BOARD_OFFSET;
y = BOARD_OFFSET + i * BLOCK_SIZE;
MoveToEx(hdc, x, y, NULL);
LineTo(hdc, x + (BOARD_SIZE - 1) * BLOCK_SIZE, y);
}
SelectObject(hdc, hOldPen);
DeleteObject(hPen);
}
void DrawStones(HDC hdc, int board[BOARD_SIZE][BOARD_SIZE])
{
HBRUSH hBrush;
int x, y, i, j;
for (i = 0; i < BOARD_SIZE; i++)
{
for (j = 0; j < BOARD_SIZE; j++)
{
if (board[i][j])
{
x = BOARD_OFFSET + j * BLOCK_SIZE - BLOCK_SIZE / 2;
y = BOARD_OFFSET + i * BLOCK_SIZE - BLOCK_SIZE / 2;
hBrush = CreateSolidBrush(board[i][j] == 1 ? RGB(0, 0, 0) : RGB(255, 255, 255));
SelectObject(hdc, hBrush);
Ellipse(hdc, x, y, x + BLOCK_SIZE, y + BLOCK_SIZE);
DeleteObject(hBrush);
}
}
}
}
```
该程序使用了一个二维数组来存储棋盘上的棋子,每次点击鼠标时根据鼠标的位置计算出对应的行列数,然后判断该位置是否已经有棋子,如果没有,则在该位置放置当前玩家的棋子,并且交换当前玩家。之后重绘棋盘即可。
需要注意的是,该程序仅是一个简单的示例程序,仅实现了基本的绘图和交互功能,实际的围棋程序还需要实现更多的功能,比如禁手规则、自我对弈等。
相关推荐
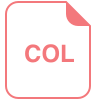
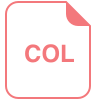
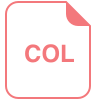
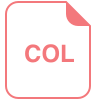
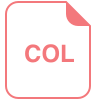










