#define _CRT_SECURE_NO_WARNINGS #include <stdio.h> #include <stdlib.h> #include <string.h> #define MAX_SIZE 100 typedef struct { int book_id; char book_name[50]; float price; } Book; typedef struct { Book books[MAX_SIZE]; int length; } BookList; void input_books(BookList* list, int n) { for (int i = 0; i < n; i++) { printf("请输入第%d本书的信息:\n", i + 1); printf("图书编号:"); scanf("%d", &list->books[i].book_id); printf("书名:"); scanf("%s", list->books[i].book_name); printf("价格:"); scanf("%f", &list->books[i].price); } list->length = n; } void display_books(BookList* list) { printf("图书表中所有图书的相关信息:\n"); for (int i = 0; i < list->length; i++) { printf("图书编号:%d\n", list->books[i].book_id); printf("书名:%s\n", list->books[i].book_name); printf("价格:%f\n", list->books[i].price); } } void insert_book(BookList* list, int pos, Book book) { if (pos < 1 || pos > list->length + 1) { printf("插入位置不合法!\n"); return; } for (int i = list->length - 1; i >= pos - 1; i--) { list->books[i + 1] = list->books[i]; } list->books[pos - 1] = book; list->length++; } void delete_book(BookList* list, int pos) { if (pos < 1 || pos > list->length) { printf("删除位置不合法!\n"); return; } for (int i = pos - 1; i < list->length - 1; i++) { list->books[i] = list->books[i + 1]; } list->length--; } int count_books(BookList* list) { return list->length; } int partition(BookList* list, int low, int high) { Book pivot = list->books[low]; while (low < high) { while (low < high && list->books[high].book_id >= pivot.book_id) high--; list->books[low] = list->books[high]; while (low < high && list->books[low].book_id <= pivot.book_id) low++; list->books[high] = list->books[low]; } list->books[low] = pivot; return low; } void quick_sort(BookList* list, int
时间: 2024-04-02 08:33:47 浏览: 16
) {
if (list->length <= 1) return;
int stack[MAX_SIZE], top = -1;
stack[++top] = 0;
stack[++top] = list->length - 1;
while (top > 0) {
int high = stack[top--];
int low = stack[top--];
int mid = partition(list, low, high);
if (low < mid - 1) {
stack[++top] = low;
stack[++top] = mid - 1;
}
if (mid + 1 < high) {
stack[++top] = mid + 1;
stack[++top] = high;
}
}
}
int main() {
BookList list;
input_books(&list, 3);
display_books(&list);
Book book = { 4, "Data Structures and Algorithms", 45.8 };
insert_book(&list, 2, book);
display_books(&list);
delete_book(&list, 3);
display_books(&list);
int count = count_books(&list);
printf("图书表中共有%d本图书。\n", count);
quick_sort(&list, count);
display_books(&list);
return 0;
}
这是一个图书管理系统的代码,实现了图书的增删查改和快速排序功能。其中Book和BookList为自定义的结构体,分别表示图书和图书表。在主函数中,先输入3本图书的信息,然后显示所有图书的信息,接着在第二个位置插入一本新书并显示所有图书的信息,再删除第三本书并显示所有图书的信息。然后统计图书数量并输出,最后对图书表进行快速排序并输出排序结果。
相关推荐
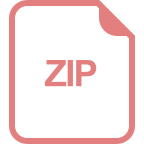
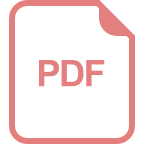
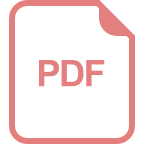
















