一个ch455实现4x5矩阵键盘灯光,包含三个数码管共24个led,每个按键只亮一个led,具体实现方法
时间: 2024-01-27 22:04:47 浏览: 121
首先,我们需要一个4x5的矩阵键盘,其中每个按键都有一个独立的引脚。假设这些引脚被连接到单片机的I/O口。
接下来,我们需要连接24个LED和3个数码管。假设这些LED和数码管被连接到单片机的另外的I/O口。
现在我们需要编写代码来控制这些LED和数码管,使它们可以显示正确的灯光。以下是代码示例:
```c
#include <reg51.h>
// 定义LED引脚
sbit LED1=P1^0;
sbit LED2=P1^1;
sbit LED3=P1^2;
// ... LED4至LED24
// 定义数码管引脚
sbit Digit1 = P2^4;
sbit Digit2 = P2^5;
sbit Digit3 = P2^6;
// 定义每个按键对应的I/O口
sbit Key11=P3^0;
sbit Key12=P3^1;
sbit Key13=P3^2;
// ... Key4至Key20
// 定义按键位置矩阵
unsigned char KeyMatrix[4][5]={
{0,1,2,3,4},
{5,6,7,8,9},
{10,11,12,13,14},
{15,16,17,18,19}
};
// 定义当前按下的按键位置
unsigned char CurrentKey = 0xFF;
// 定义LED状态数组
unsigned char LEDState[24]={
0,0,0,0,0,0,0,0,0,0,0,0,
0,0,0,0,0,0,0,0,0,0,0,0
};
// 定义数码管显示数组
unsigned char DisplayData[3]={
0,0,0
};
// 数码管显示函数
void Display() {
static unsigned char i = 0;
Digit1 = 1;
Digit2 = 1;
Digit3 = 1;
switch(i) {
case 0:
Digit1 = 0;
P0 = DisplayData[i];
break;
case 1:
Digit2 = 0;
P0 = DisplayData[i];
break;
case 2:
Digit3 = 0;
P0 = DisplayData[i];
break;
}
i++;
if (i >= 3) i = 0;
}
// 更新LED状态函数
void UpdateLED() {
// 关闭所有LED灯
LED1 = 0;
LED2 = 0;
LED3 = 0;
// ... LED4至LED24 = 0
// 打开当前按下的按键对应的LED灯
if (CurrentKey != 0xFF) {
LEDState[CurrentKey] = 1;
}
for (int i = 0; i < 24; i++) {
if (LEDState[i] == 1) {
switch(i) {
case 0:
LED1 = 1;
break;
case 1:
LED2 = 1;
break;
case 2:
LED3 = 1;
break;
// ... LED4至LED24
}
}
}
}
// 主函数
void main() {
while(1) {
// 扫描按键
for (unsigned char i = 0; i < 4; i++) {
for (unsigned char j = 0; j < 5; j++) {
if (KeyMatrix[i][j] != CurrentKey) {
if (KeyMatrix[i][j] == 0xFF) {
// 没有按键按下
continue;
}
// 检测到按键按下
CurrentKey = KeyMatrix[i][j];
}
}
}
// 更新LED状态
UpdateLED();
// 显示数码管数字
DisplayData[0] = CurrentKey % 10;
DisplayData[1] = (CurrentKey / 10) % 10;
DisplayData[2] = (CurrentKey / 100) % 10;
Display();
}
}
```
在上述代码中,我们首先定义了LED引脚、数码管引脚和按键位置矩阵等变量。然后我们使用一个双重循环扫描按键矩阵,检测当前是否有按键按下,并记录下当前按下的按键位置。在每次循环中,我们使用UpdateLED()函数来更新LED的状态,根据当前按下的按键位置来控制LED灯的亮灭。在UpdateLED()函数中,我们使用LEDState数组来记录每个LED灯的状态,使用LED1至LED24来控制每个LED灯的亮灭。同时,我们使用Display()函数来显示数码管的数字。在Display()函数中,我们使用DisplayData数组来记录需要显示的数字,使用Digit1、Digit2和Digit3来控制数码管的显示位。
以上就是一个简单的4x5矩阵键盘灯光的实现方法,希望对你有所帮助。
阅读全文
相关推荐
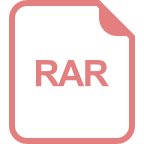
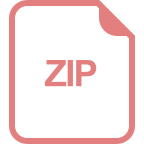













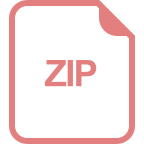