利用Openmv完成蓝色长方体表面的黄色圆形的识别,生成代码
时间: 2023-12-14 21:36:20 浏览: 34
以下是使用OpenMV识别蓝色长方体表面的黄色圆形的代码。请确保已经安装了OpenMV IDE并正确连接了OpenMV相机。
```python
import sensor, image, time
# 设置颜色追踪阈值
blue_threshold = (30, 100, -30, 30, -30, 30)
yellow_threshold = (60, 100, -40, 40, -0, 40)
# 初始化摄像头
sensor.reset()
sensor.set_pixformat(sensor.RGB565)
sensor.set_framesize(sensor.QVGA)
sensor.skip_frames(time = 2000)
# 初始化显示屏
lcd = None
try:
import lcd160cr
lcd = lcd160cr.LCD160CR('X')
lcd.set_orient(lcd160cr.PORTRAIT)
lcd.erase()
except:
print("LCD screen not found.")
# 设置图像缩放比例
scale = 1.5
while True:
# 获取图像
img = sensor.snapshot()
# 图像缩放
img = img.resize(int(img.width() / scale), int(img.height() / scale))
# 颜色追踪
blue_blobs = img.find_blobs([blue_threshold], pixels_threshold=100, area_threshold=100, merge=True)
yellow_blobs = img.find_blobs([yellow_threshold], pixels_threshold=100, area_threshold=100, merge=True)
# 绘制识别结果
if blue_blobs:
for b in blue_blobs:
img.draw_rectangle(b.rect(), color=(255, 0, 0))
img.draw_string(b.x()+10, b.y()-10, "Blue Box", color=(255, 0, 0), scale=2)
if lcd:
lcd.erase()
lcd.set_pen(lcd.rgb(255, 0, 0), lcd.rgb(0, 0, 0))
lcd.rect(int(b.x() * scale), int(b.y() * scale), int(b.w() * scale), int(b.h() * scale))
lcd.set_pen(lcd.rgb(255, 255, 255), lcd.rgb(0, 0, 0))
lcd.set_text_pos(0, 0)
lcd.write("Blue Box")
if yellow_blobs:
for b in yellow_blobs:
img.draw_circle(b.cx(), b.cy(), int(b.r()), color=(255, 255, 0))
img.draw_string(b.x()+10, b.y()-10, "Yellow Circle", color=(255, 255, 0), scale=2)
if lcd:
lcd.erase()
lcd.set_pen(lcd.rgb(255, 255, 0), lcd.rgb(0, 0, 0))
lcd.circle(int(b.cx() * scale), int(b.cy() * scale), int(b.r() * scale))
lcd.set_pen(lcd.rgb(255, 255, 255), lcd.rgb(0, 0, 0))
lcd.set_text_pos(0, 0)
lcd.write("Yellow Circle")
# 显示识别结果
img = img.resize(int(img.width() * scale), int(img.height() * scale))
img.compress(quality=90)
if lcd:
lcd.set_orient(lcd160cr.LANDSCAPE)
lcd.display(img)
lcd.set_orient(lcd160cr.PORTRAIT)
# 延时等待
time.sleep(100)
```
代码解释:
1. 首先定义了两个颜色阈值:`blue_threshold` 和 `yellow_threshold`,用于识别蓝色长方体和黄色圆形。
2. 初始化摄像头和显示屏。
3. 设置图像缩放比例,可以加快图像处理速度。
4. 进入主循环,在每次循环中获取图像并进行颜色追踪。
5. 如果发现蓝色长方体或黄色圆形,就在图像上绘制对应的识别框和文字,并在显示屏上显示识别结果。
6. 最后延时等待,等待下一次循环。
注意事项:
1. 颜色阈值需要根据实际情况进行调整,可以通过OpenMV IDE中的颜色追踪工具进行调试。
2. 如果没有连接显示屏,可以将显示屏相关的代码注释掉,只使用串口或者USB进行输出。
相关推荐
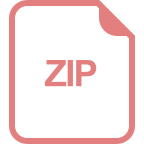
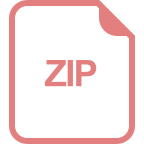














