实现3个类:Storage、Counter和Printer。 Storage类应存储整数。 Counter应创建线程,线程从0开始计数(0,1,2,3…)并将每个值存储到Storage类中。 Printer类应创建一个线程,线程读取Storage类中的值并打印值。编写程序创建Storage类的实例,并创建一个Counter对象和Printer对象操作此实例。
时间: 2024-02-13 17:04:19 浏览: 53
下面是一个简单的实现,实现了Storage、Counter和Printer这三个类的功能:
```java
public class Storage {
private int value;
public synchronized int getValue() {
return value;
}
public synchronized void setValue(int value) {
this.value = value;
}
}
public class Counter implements Runnable {
private Storage storage;
public Counter(Storage storage) {
this.storage = storage;
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
storage.setValue(i);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Printer implements Runnable {
private Storage storage;
public Printer(Storage storage) {
this.storage = storage;
}
@Override
public void run() {
for (int i = 0; i < 10; i++) {
System.out.println("Value: " + storage.getValue());
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
public class Main {
public static void main(String[] args) {
Storage storage = new Storage();
Counter counter = new Counter(storage);
Printer printer = new Printer(storage);
Thread counterThread = new Thread(counter);
Thread printerThread = new Thread(printer);
counterThread.start();
printerThread.start();
try {
counterThread.join();
printerThread.join();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
```
在这个例子中,Storage类存储一个整数值,并提供了getValue()和setValue()方法来读取和设置这个值。Counter类实现了Runnable接口,创建了一个线程来计数并将计数值存储到Storage类中。Printer类也实现了Runnable接口,创建了一个线程来读取Storage类中的值并打印它们。Main类创建了Storage、Counter和Printer这三个对象,并启动了它们的线程。最后,Main类等待线程完成执行。
相关推荐
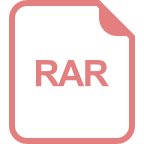
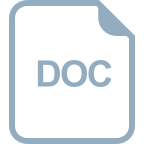














