linux 使用POSIX 消息队列实现线程间通信的例子
时间: 2023-05-25 13:03:56 浏览: 137
以下是一个使用 POSIX 消息队列实现线程间通信的例子:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <pthread.h>
#include <mqueue.h>
#define MSG_SIZE 256
#define MAX_MSG 10
mqd_t mqd;
pthread_t tid[2];
pthread_attr_t attr;
// 线程1:发送消息
void *send_func(void *arg) {
char msg[MSG_SIZE];
int i;
for (i = 0; i < MAX_MSG; i++) {
memset(msg, 0, MSG_SIZE);
sprintf(msg, "Message %d from thread 1", i);
if (mq_send(mqd, msg, strlen(msg) + 1, 0) == -1) {
perror("mq_send");
exit(1);
}
printf("Thread 1 sent: %s\n", msg);
sleep(1);
}
}
// 线程2:接收消息
void *recv_func(void *arg) {
char msg[MSG_SIZE];
unsigned int prio;
int i;
for (i = 0; i < MAX_MSG; i++) {
memset(msg, 0, MSG_SIZE);
if (mq_receive(mqd, msg, MSG_SIZE, &prio) == -1) {
perror("mq_receive");
exit(1);
}
printf("Thread 2 received: %s\n", msg);
sleep(1);
}
}
int main() {
struct mq_attr attr;
attr.mq_flags = 0;
attr.mq_maxmsg = 10;
attr.mq_msgsize = MSG_SIZE;
attr.mq_curmsgs = 0;
if ((mqd = mq_open("/test_mq", O_CREAT | O_RDWR, S_IRUSR | S_IWUSR, &attr)) == -1) {
perror("mq_open");
exit(1);
}
if (pthread_attr_init(&attr) != 0) {
perror("pthread_attr_init");
exit(1);
}
if (pthread_create(&tid[0], &attr, send_func, NULL) != 0) {
perror("pthread_create");
exit(1);
}
if (pthread_create(&tid[1], &attr, recv_func, NULL) != 0) {
perror("pthread_create");
exit(1);
}
if (pthread_join(tid[0], NULL) != 0) {
perror("pthread_join");
exit(1);
}
if (pthread_join(tid[1], NULL) != 0) {
perror("pthread_join");
exit(1);
}
if (mq_close(mqd) == -1) {
perror("mq_close");
exit(1);
}
if (mq_unlink("/test_mq") == -1) {
perror("mq_unlink");
exit(1);
}
return 0;
}
```
该程序创建了一个 POSIX 消息队列 `/test_mq`,其中维护了最大消息数为 10,每条消息为 256 字节。程序启动两个线程,一个用于发送消息,一个用于接收消息,它们都可以同时操作消息队列。发送线程每秒钟向队列中发送一条消息,接收线程每秒钟从队列中接收一条消息并打印出来。程序使用 `pthread_create()` 创建线程,使用 `pthread_join()` 以等待线程完成,使用 `mq_send()` 发送消息,使用 `mq_receive()` 接收消息。最后程序清理了 POSIX 消息队列。
阅读全文
相关推荐
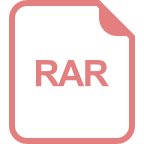
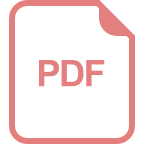
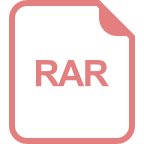















