蚁群算法C语言实现
时间: 2023-08-10 13:06:02 浏览: 208
蚁群算法(Ant Colony Optimization,ACO)是一种模拟蚂蚁在寻找食物过程中的行为规律,从而优化问题求解的启发式算法。
以下是一个简单的蚁群算法的C语言实现,用于求解旅行商问题(TSP):
```
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#include <time.h>
#define N 10 // 城市数量
#define M 100 // 蚂蚁数量
#define ALPHA 1 // 信息素重要程度因子
#define BETA 5 // 启发函数重要程度因子
#define RHO 0.5 // 信息素挥发因子
#define Q 100 // 常系数
#define MAX_T 1000 // 迭代次数
double distance[N][N]; // 城市距离矩阵
double pheromone[N][N]; // 信息素矩阵
int ants[M][N]; // 蚂蚁走过的路径
double length[M]; // 蚂蚁走过的路径长度
int best_ant[N]; // 最优路径
double best_length = 1e9; // 最优路径长度
double rand01() {
return (double)rand() / RAND_MAX;
}
int rand_int(int n) {
return rand() % n;
}
// 计算两个城市之间的距离
double city_distance(int city1, int city2) {
return distance[city1][city2];
}
// 初始化城市距离矩阵和信息素矩阵
void init() {
srand((unsigned)time(NULL));
int i, j;
for (i = 0; i < N; i++) {
for (j = i; j < N; j++) {
if (i == j) {
distance[i][j] = 0;
pheromone[i][j] = 0;
} else {
distance[i][j] = distance[j][i] = rand01();
pheromone[i][j] = pheromone[j][i] = 0.01;
}
}
}
}
// 蚂蚁选择下一个城市
int select_next_city(int ant, int current_city) {
int i;
double p[N], sum = 0;
for (i = 0; i < N; i++) {
if (ants[ant][i] == -1) {
double eta = 1.0 / distance[current_city][i];
p[i] = pow(pheromone[current_city][i], ALPHA) * pow(eta, BETA);
sum += p[i];
}
}
if (sum == 0) return -1;
double r = rand01() * sum;
for (i = 0; i < N; i++) {
if (ants[ant][i] == -1) {
r -= p[i];
if (r < 0) return i;
}
}
return -1;
}
// 蚂蚁进行一次完整的旅行
void ant_travel(int ant) {
int i;
for (i = 0; i < N; i++) {
ants[ant][i] = -1;
}
int current_city = rand_int(N);
ants[ant][current_city] = 1;
length[ant] = 0;
for (i = 1; i < N; i++) {
int next_city = select_next_city(ant, current_city);
if (next_city == -1) break;
ants[ant][next_city] = i + 1;
length[ant] += city_distance(current_city, next_city);
current_city = next_city;
}
length[ant] += city_distance(current_city, ants[ant][0] - 1]);
if (length[ant] < best_length) {
best_length = length[ant];
for (i = 0; i < N; i++) {
best_ant[i] = ants[ant][i];
}
}
}
// 更新信息素矩阵
void update_pheromone() {
int i, j;
for (i = 0; i < N; i++) {
for (j = i; j < N; j++) {
pheromone[i][j] *= (1 - RHO);
pheromone[j][i] = pheromone[i][j];
}
}
for (i = 0; i < M; i++) {
double delta_pheromone = Q / length[i];
for (j = 0; j < N; j++) {
int city1 = ants[i][j];
int city2 = ants[i][(j + 1) % N];
pheromone[city1 - 1][city2 - 1] += delta_pheromone;
pheromone[city2 - 1][city1 - 1] = pheromone[city1 - 1][city2 - 1];
}
}
}
int main() {
init();
int t;
for (t = 0; t < MAX_T; t++) {
int i;
for (i = 0; i < M; i++) {
ant_travel(i);
}
update_pheromone();
}
printf("Best length: %lf\n", best_length);
printf("Best path: ");
int i;
for (i = 0; i < N; i++) {
printf("%d ", best_ant[i]);
}
printf("\n");
return 0;
}
```
该实现中,首先定义了几个常数,包括城市数量、蚂蚁数量、信息素重要程度因子、启发函数重要程度因子、信息素挥发因子等。然后定义了一些函数,包括计算两个城市之间的距离、初始化城市距离矩阵和信息素矩阵、蚂蚁选择下一个城市、蚂蚁进行一次完整的旅行、更新信息素矩阵等。最后在主函数中进行迭代,不断让蚂蚁进行旅行,并更新信息素矩阵。迭代结束后输出最优路径和最优路径长度。
该实现中的算法并不是最优的,仅供参考。实际应用中需要根据具体问题进行调整和优化。
阅读全文
相关推荐
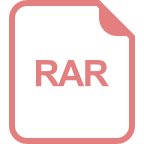
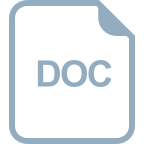
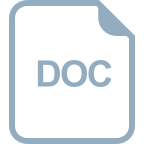
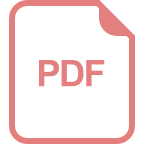
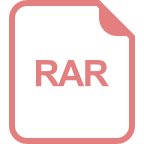
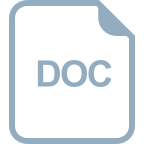





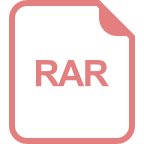
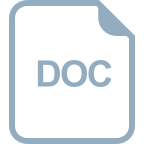
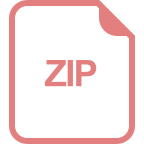
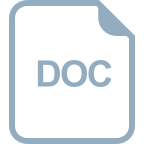
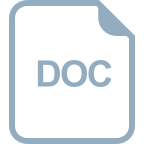
