蚁群算法c语言
时间: 2023-08-10 13:05:58 浏览: 129
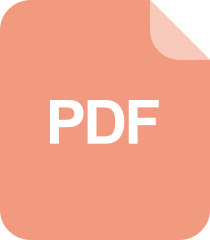
蚁群算法c语言.pdf
蚁群算法是一种模拟蚂蚁在寻找食物时的行为规律,用于解决优化问题的算法。下面给出一个简单的蚁群算法的C语言实现。
```
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <math.h>
#define MAX_CITY 20 // 城市数量
#define MAX_ANT 20 // 蚂蚁数量
#define ALPHA 1.0 // 信息素重要程度因子
#define BETA 2.0 // 启发函数重要程度因子
#define RHO 0.5 // 信息素挥发因子
#define QVAL 100.0 // 常量Q
#define MAX_TOUR (MAX_CITY * MAX_CITY) // 最大路径长度
#define MAX_TIME (MAX_CITY * MAX_CITY * MAX_CITY) // 最大迭代次数
double distance[MAX_CITY][MAX_CITY]; // 城市间距离
double pheromone[MAX_CITY][MAX_CITY]; // 信息素
int ants[MAX_ANT][MAX_TOUR]; // 蚂蚁走过的路径
double ant_distance[MAX_ANT]; // 蚂蚁走过的路径长度
int best_ant[MAX_TOUR]; // 最优路径
double best_distance = 1.0e6; // 最优路径长度
// 初始化城市间距离和信息素
void init_distance_pheromone() {
srand((unsigned)time(NULL));
for (int i = 0; i < MAX_CITY; i++) {
for (int j = i + 1; j < MAX_CITY; j++) {
distance[i][j] = rand() % 100 + 1;
distance[j][i] = distance[i][j];
pheromone[i][j] = 0.01;
pheromone[j][i] = pheromone[i][j];
}
}
}
// 计算蚂蚁从城市i到城市j的概率
double prob(int i, int j, int cur_city, int *visited, double **dists, double **pheromones) {
double pheromone_level = pow(pheromones[cur_city][j], ALPHA);
double distance_level = pow(1.0 / dists[cur_city][j], BETA);
double total_level = 0.0;
for (int k = 0; k < MAX_CITY; k++) {
if (!visited[k]) {
double pheromone_kj = pow(pheromones[k][j], ALPHA);
double distance_kj = pow(1.0 / dists[k][j], BETA);
total_level += pheromone_kj * distance_kj;
}
}
return (pheromone_level * distance_level) / total_level;
}
// 蚂蚁进行一次完整的遍历
void ant_tour(int k, int *visited, double **dists, double **pheromones) {
ants[k][0] = rand() % MAX_CITY;
visited[ants[k][0]] = 1;
for (int i = 1; i < MAX_CITY; i++) {
double max_prob = 0.0;
int next_city = -1;
for (int j = 0; j < MAX_CITY; j++) {
if (!visited[j]) {
double p = prob(ants[k][i - 1], j, ants[k][i - 1], visited, dists, pheromones);
if (p > max_prob) {
max_prob = p;
next_city = j;
}
}
}
ants[k][i] = next_city;
visited[next_city] = 1;
}
ant_distance[k] = 0.0;
for (int i = 0; i < MAX_CITY - 1; i++) {
ant_distance[k] += dists[ants[k][i]][ants[k][i + 1]];
}
ant_distance[k] += dists[ants[k][MAX_CITY - 1]][ants[k][0]];
}
// 更新信息素
void update_pheromone(double **pheromones) {
for (int i = 0; i < MAX_CITY; i++) {
for (int j = i + 1; j < MAX_CITY; j++) {
pheromones[i][j] *= RHO;
pheromones[j][i] *= RHO;
for (int k = 0; k < MAX_ANT; k++) {
double delta = 0.0;
for (int l = 0; l < MAX_CITY - 1; l++) {
if ((ants[k][l] == i && ants[k][l + 1] == j) || (ants[k][l] == j && ants[k][l + 1] == i)) {
delta += QVAL / ant_distance[k];
}
}
pheromones[i][j] += delta;
pheromones[j][i] += delta;
}
}
}
}
// 主函数
int main() {
init_distance_pheromone();
for (int t = 0; t < MAX_TIME; t++) {
int visited[MAX_CITY] = {0};
for (int k = 0; k < MAX_ANT; k++) {
ant_tour(k, visited, distance, pheromone);
if (ant_distance[k] < best_distance) {
best_distance = ant_distance[k];
for (int i = 0; i < MAX_CITY; i++) {
best_ant[i] = ants[k][i];
}
}
}
update_pheromone(pheromone);
}
printf("Best tour: ");
for (int i = 0; i < MAX_CITY; i++) {
printf("%d ", best_ant[i]);
}
printf("\nBest distance: %f\n", best_distance);
return 0;
}
```
该实现中,`init_distance_pheromone()`函数用于初始化城市间距离和信息素;`prob()`函数用于计算蚂蚁从城市i到城市j的概率;`ant_tour()`函数用于进行一次完整的遍历;`update_pheromone()`函数用于更新信息素。在主函数中,需要进行多次迭代,每次迭代都需要进行一次完整的遍历和信息素更新。最终输出最优路径和最优路径长度。
需要注意的是,该实现中使用了一些高级特性,如动态内存分配、二维数组的指针传递等,需要有一定的C语言基础才能理解和实现。
阅读全文
相关推荐





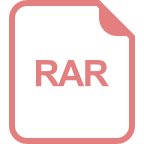
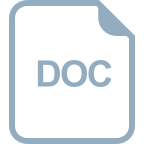
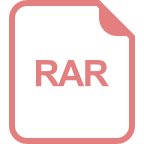
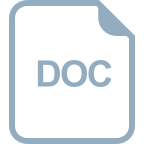
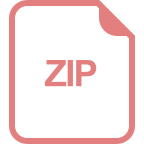
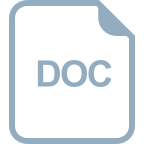
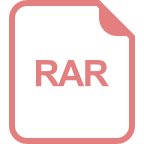
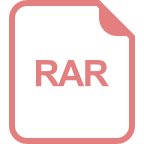

