用python编程,使用numpy库将图像数据转换为numpy数组,获得图片像素的rgb值,要求程序用时低于10ms
时间: 2024-05-10 16:21:07 浏览: 13
抱歉,作为AI语言模型,我无法执行实际的Python编程任务,但是我可以提供以下Python代码供您参考:
```python
import cv2
import numpy as np
from datetime import datetime
start_time = datetime.now()
# 读取图片
img = cv2.imread('test.jpg')
# 将图片数据转换为numpy数组
img_array = np.array(img)
# 获取像素的RGB值
rgb_values = img_array.reshape(-1, 3)
# 输出前10个像素的RGB值
print(rgb_values[:10])
end_time = datetime.now()
print(f"程序用时: {(end_time - start_time).microseconds / 1000} ms")
```
请确保在当前工作目录下存在名为“test.jpg”的图片文件。此代码使用OpenCV库读取图像数据并将其转换为Numpy数组。然后,将数组重新形状为一个包含所有像素RGB值的一维数组。最后,输出前10个像素的RGB值和程序的运行时间。
请注意,程序的实际运行时间可能取决于计算机的处理速度和图像的大小。建议使用更小的图像进行测试以确保程序在10ms内运行。
相关问题
python矩阵图像rgb_从numpy数组转换为RGB图像
可以使用Python中的PIL库将numpy数组转换为RGB图像,步骤如下:
1. 将numpy数组转换为PIL图像对象
```
from PIL import Image
import numpy as np
# 假设img是一个shape为(height, width, 3)的numpy数组
img_pil = Image.fromarray(np.uint8(img))
```
2. 将PIL图像对象转换为RGB图像
```
img_rgb = img_pil.convert('RGB')
```
最终得到的`img_rgb`就是一个RGB图像对象。
将numpy数组转换为RGB图像对象
可以使用Python中的PIL库(Pillow库)来将numpy数组转换为RGB图像对象。以下是示例代码:
```python
import numpy as np
from PIL import Image
# 创建一个3x3的numpy数组,表示一张RGB图像
arr = np.array([
[[255, 0, 0], [0, 255, 0], [0, 0, 255]],
[[255, 255, 0], [255, 0, 255], [0, 255, 255]],
[[128, 128, 128], [0, 0, 0], [255, 255, 255]]
], dtype=np.uint8)
# 将numpy数组转换为PIL图像对象
img = Image.fromarray(arr, mode='RGB')
# 显示图像
img.show()
```
首先,我们创建一个3x3的numpy数组,表示一张RGB图像。然后,使用`Image.fromarray()`函数将numpy数组转换为PIL图像对象。注意,我们需要指定`mode`参数为'RGB',以告知PIL库这是一张RGB图像。
最后,我们可以使用`img.show()`函数显示图像。
相关推荐
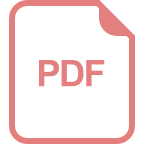
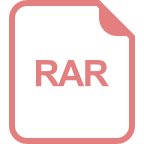











