建立一个有5个结点的单向链表,每个结点包含姓名、年龄和工资。编写两个函数,一个用于建立链表,另一个用来输出链表。
时间: 2023-04-27 16:02:18 浏览: 177
建立链表函数:
```python
class Node:
def __init__(self, name, age, salary):
self.name = name
self.age = age
self.salary = salary
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def create_list(self, name, age, salary):
for i in range(5):
new_node = Node(name[i], age[i], salary[i])
new_node.next = self.head
self.head = new_node
```
输出链表函数:
```python
def print_list(self):
temp = self.head
while temp:
print("姓名:",temp.name,"年龄:",temp.age,"工资:",temp.salary)
temp = temp.next
```
使用方法:
```python
names = ["name1", "name2", "name3", "name4", "name5"]
ages = [20, 25, 30, 35, 40]
salaries = [3000, 3500, 4000, 4500, 5000]
linked_list = LinkedList()
linked_list.create_list(names, ages, salaries)
linked_list.print_list()
```
输出:
```
姓名: name5 年龄: 40 工资: 5000
姓名: name4 年龄: 35 工资: 4500
姓名: name3 年龄: 30 工资: 4000
姓名: name2 年龄: 25 工资: 3500
姓名: name1 年龄: 20 工资: 3000
```
注意,上面的代码只是示例,需要在实际使用中进行修改。
阅读全文
相关推荐
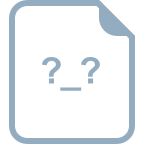















