python创建线程能否将一个对象作为参数传递进去,如果可以,具体步骤是什么
时间: 2024-05-03 15:20:51 浏览: 9
可以将一个对象作为参数传递给Python创建线程。具体步骤是使用threading模块中的Thread类创建线程,然后使用args参数将对象传递给线程。例如:
```
import threading
class MyThread(threading.Thread):
def __init__(self, my_obj):
threading.Thread.__init__(self)
self.my_obj = my_obj
def run(self):
# do something with my_obj
my_obj = MyObject()
my_thread = MyThread(my_obj)
my_thread.start()
```
在这个例子中,MyObject是一个自定义的对象,MyThread是一个继承了Thread类的自定义线程类。我们将my_obj作为参数传递给了MyThread类的构造函数,并在run方法中使用了该对象。最后,我们启动了线程并让它开始执行。
相关问题
如果线程中函数返回结果 ,在线程执行完成后 怎么获取这个结果 python语句实现
在 Python 中,如果想要在线程中返回结果并在主线程中获取这个结果,可以使用 threading 模块中的 Thread 类和 Queue 类。具体实现步骤如下:
1. 定义一个函数,这个函数将会在子线程中执行,并返回一个结果。
2. 创建一个 Queue 对象,这个对象将会用于在主线程中获取子线程返回的结果。
3. 创建一个 Thread 对象,将函数和 Queue 对象作为参数传递给 Thread 对象。
4. 启动线程。
5. 在主线程中从 Queue 对象中获取子线程返回的结果。
下面是一个示例代码,演示了如何在线程中返回结果并在主线程中获取这个结果:
```python
import threading
import queue
def worker(q):
# 在子线程中执行的函数
result = 42
q.put(result) # 将结果放入队列中
# 创建一个队列,用于在主线程中获取子线程返回的结果
q = queue.Queue()
# 创建一个线程,并将函数和队列对象作为参数传递给线程对象
t = threading.Thread(target=worker, args=(q,))
# 启动线程
t.start()
# 在主线程中从队列中获取子线程返回的结果
result = q.get()
print(result) # 打印结果
```
在上面的代码中,worker() 函数将会在子线程中执行,并返回一个结果。在主线程中,我们创建了一个队列对象 q,用于获取子线程返回的结果。然后,我们创建了一个线程对象 t,并将 worker() 函数和队列对象 q 作为参数传递给线程对象。最后,我们启动线程,并在主线程中从队列对象 q 中获取子线程返回的结果,并打印这个结果。
python多线程使用
在Python中,多线程是一种并发编程技术,允许一个程序同时执行多个任务,每个任务在单独的线程中运行。使用Python的内置`threading`模块可以轻松地创建和管理线程。以下是使用Python多线程的基本步骤:
1. 导入`threading`模块:这是开始多线程编程的第一步。
```python
import threading
```
2. 定义线程函数(target):你需要为每个线程定义一个函数,这个函数将在新线程中执行。
```python
def worker_function(arg):
# 线程执行的代码
pass
```
3. 创建线程对象:使用`Thread`类实例化一个线程,将定义的函数作为参数传递。
```python
thread = threading.Thread(target=worker_function, args=(arg,))
```
4. 启动线程:调用`start()`方法启动线程,此时线程会在后台运行。
```python
thread.start()
```
5. 等待线程完成:如果你需要等待线程结束,可以使用`join()`方法。
```python
thread.join() # 如果你想让主线程等待这个线程结束
```
6. 错误处理:线程可能遇到异常,使用`threading`模块提供的异常处理器来捕获并处理。
```python
try:
thread.start()
except Exception as e:
print(f"Error in thread: {e}")
```
需要注意的是,Python中的全局解释器锁(GIL)限制了同一时间只能有一个线程在执行Python字节码。这意味着多线程并不能真正实现并行计算,但在IO密集型任务中仍然很有用,比如网络请求、文件读写等。
相关推荐
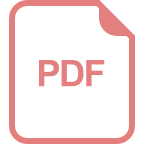
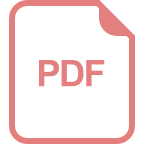
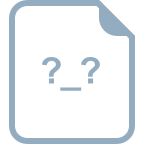












