优化这段代码import javax.swing.*; import java.awt.*; import java.awt.event.*; public class TemperatureConverter implements ActionListener { private JFrame frame; private JPanel panel; private JLabel Label1,Label2; private JTextField Field1,Field2; private JButton Button1,Button2,Button3; public TemperatureConverter() { frame = new JFrame("温度转换"); f.setSize ( 400,200 ) ; f.setVisible ( true ) ; f.setLocationRelativeTo ( null ) ; panel = new JPanel(); Label1 = new JLabel("摄氏度:"); Label2 = new JLabel("华氏度:"); Field1 = new JTextField(10); Field2 = new JTextField(10); Button1 = new Button("摄氏度 → 华氏度"); Button2 = new Button("华氏度 → 摄氏度"); Button3 = new Button("清空"); panel.add(Label1); panel.add(Label2); panel.add(Field1); panel.add(Field2); panel.add(Button1); panel.add(Button2); panel.add(Button3); Button1.addActionListener(this); Button2.addActionListener(this); Button3.addActionListener(this); public void actionPerformed(ActionEvent e) { if (e.getSource() == Button1) { double value = Double.parseDouble(Field1.getText()); double result = (value * 9 / 5) + 32; Field1.setText(String.format("%.2f", result)); } if (e.getSource() == Button2) { double value = Double.parseDouble(Field2.getText()); double result = (value - 32) * 5 / 9; Field1.setText(String.format("%.2f", result)); } if(e.getActionCommand().equals("清空") ) { Field1.setText(null); Field2.setText(null); } } } } public static void main(String[] args) { new TemperatureConverter(); }
时间: 2023-12-06 18:42:09 浏览: 36
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class TemperatureConverter implements ActionListener {
private JFrame frame;
private JPanel panel;
private JLabel label1, label2;
private JTextField field1, field2;
private JButton button1, button2, button3;
public TemperatureConverter() {
frame = new JFrame("温度转换");
frame.setSize(400, 200);
frame.setVisible(true);
frame.setLocationRelativeTo(null);
panel = new JPanel();
label1 = new JLabel("摄氏度:");
label2 = new JLabel("华氏度:");
field1 = new JTextField(10);
field2 = new JTextField(10);
button1 = new JButton("摄氏度 → 华氏度");
button2 = new JButton("华氏度 → 摄氏度");
button3 = new JButton("清空");
panel.add(label1);
panel.add(field1);
panel.add(label2);
panel.add(field2);
panel.add(button1);
panel.add(button2);
panel.add(button3);
button1.addActionListener(this);
button2.addActionListener(this);
button3.addActionListener(this);
frame.add(panel);
}
public void actionPerformed(ActionEvent e) {
if (e.getSource() == button1) {
try {
double value = Double.parseDouble(field1.getText());
double result = (value * 9 / 5) + 32;
field2.setText(String.format("%.2f", result));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(frame, "请输入有效数字!");
}
} else if (e.getSource() == button2) {
try {
double value = Double.parseDouble(field2.getText());
double result = (value - 32) * 5 / 9;
field1.setText(String.format("%.2f", result));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(frame, "请输入有效数字!");
}
} else if (e.getSource() == button3) {
field1.setText("");
field2.setText("");
}
}
public static void main(String[] args) {
new TemperatureConverter();
}
}
- 将 Button 改成 JButton。
- 添加异常处理,以防用户输入无效数字。
- 将清空操作的代码改为清空两个文本框。
相关推荐
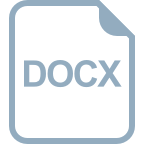
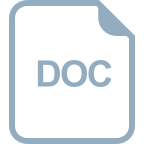
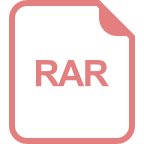
















