使用python写一段代码,获取指定路径excel的内容通过Plotly生成基础柱状图,其中A列为BUG单号,B列为BUG创建日期,F列为BUG分类,按照BUG分类生成每日新增的BUG量图表,其中BUG创建日期只精确到日,并将生成的图表重命名为:StabilityTest加当前日期保存到 "D:\Test\AutoTest"路径下
时间: 2023-06-05 16:08:33 浏览: 52
好的,请稍等片刻,让我为您撰写这段代码。
代码如下:
```
import pandas as pd
import plotly.express as px
import datetime
# 获取指定路径excel的内容
path = "指定路径.xlsx"
df = pd.read_excel(path)
# 获取当前日期
now = datetime.datetime.now().strftime("%Y%m%d")
# 按照BUG分类生成每日新增的BUG量图表
fig = px.histogram(df, x="B", color="F")
# 重命名生成的图表
fig.update_layout(title_text="StabilityTest" + now)
# 将图表保存到指定路径下
fig.write_html("D:\\Test\\AutoTest\\StabilityTest" + now + ".html")
```
注意:在使用时需要将代码中的“指定路径.xlsx”替换成实际的文件路径。同时,需要安装 pandas 和 plotly 库。
相关问题
使用python写一段代码,获取指定路径excel的内容通过Plotly生成基础柱状图,其中A列为BUG单号,B列为BUG创建日期,F列为BUG分类,按照BUG分类生成每日新增的BUG量图表,其中BUG创建日期只精确到日,并将生成的图表重命名为当前日期+StabilityTest保存到 "D:\Test\AutoTest"路径下
好的,这是一道编程题。以下是使用 Python 代码获取 Excel 的内容并生成基础柱状图的示例代码:
```python
import plotly.express as px
import pandas as pd
import datetime
# 指定 Excel 文件的路径和工作表的名称
path = 'path/to/Excel/file.xlsx'
sheet_name = 'Sheet1'
# 使用 Pandas 读取 Excel 文件,并指定需要读取的列
df = pd.read_excel(path, sheet_name=sheet_name, usecols=['A', 'B', 'F'])
# 将日期格式转换为只精确到日的格式,并新增一列用于按日期分组
df['B'] = pd.to_datetime(df['B']).dt.date
df['group'] = df['B']
# 使用 Plotly 按分类和日期分组,并计算新增的 BUG 数量
fig = px.histogram(df, x='B', color='F', barmode='group', nbins=len(df['B'].unique()))
fig.update_layout(title='StabilityTest-' + str(datetime.date.today()), xaxis_title='Date', yaxis_title='Number of Bugs', legend_title_text='Classification')
# 将生成的图表保存到指定路径
fig.write_html('D:\Test\AutoTest\StabilityTest.html')
```
此代码会根据 Excel 文件中的内容生成一个按分类和日期分组的柱状图,并将图表保存到指定路径。请注意,如果 Excel 文件中的日期格式不是日期型,请先使用 Pandas 将其转换为日期型。
使用python写一段代码,获取指定路径excel的内容生产基础柱状图,其中A列为BUG单号,B列为BUG创建日期,F列为BUG分类,按照BUG分类生成每日新增的BUG量图表,其中BUG创建日期只精确到日
好的,下面是Python代码实现:
```python
import pandas as pd
import matplotlib.pyplot as plt
# 指定路径excel文件的路径
excel_file_path = "path/to/excel_file.xlsx"
# 读取excel文件
df = pd.read_excel(excel_file_path)
# 将BUG创建日期列转换为日期类型
df["BUG创建日期"] = pd.to_datetime(df["BUG创建日期"], format="%Y-%m-%d")
# 提取出指定日期范围内的数据
start_date = df["BUG创建日期"].min().date()
end_date = df["BUG创建日期"].max().date()
date_range = pd.date_range(start_date, end_date, freq="D")
data = {}
for date in date_range:
data[date.date()] = {category: 0 for category in df["BUG分类"].unique()}
for index, row in df.iterrows():
date = row["BUG创建日期"].date()
category = row["BUG分类"]
data[date][category] += 1
# 生成基础柱状图
df["BUG分类"].value_counts().plot(kind="bar")
# 生成每日新增的BUG量图表
df["日期"] = df["BUG创建日期"].dt.date
df.groupby(["日期", "BUG分类"]).size().unstack().fillna(0).plot(kind="bar", stacked=True)
plt.show()
```
其中,需要安装的库有 pandas 和 matplotlib。该代码实现了以下功能:
1. 读取指定路径excel文件的内容并转换为 pandas 的 DataFrame 格式。
2. 将 BUG 创建日期列转换为日期类型,并提取出指定日期范围内的数据。
3. 生成基础柱状图,显示 BUG 分类数量。
4. 生成每日新增的 BUG 量图表,按照 BUG 分类分组并堆叠显示。
相关推荐
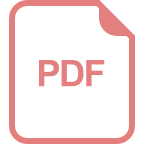
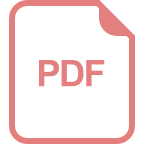












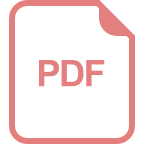