C++实现RSA,参数p,q选取三位的十进制数
时间: 2024-02-24 15:55:24 浏览: 21
好的,以下是一个简单的C++实现RSA的示例代码,其中p和q均选取了三位的素数:
```c++
#include <iostream>
#include <cmath>
// 计算最大公约数
int gcd(int a, int b) {
if (b == 0) {
return a;
} else {
return gcd(b, a % b);
}
}
// 计算模反元素
int mod_inverse(int a, int m) {
int m0 = m, t, q;
int x0 = 0, x1 = 1;
if (m == 1) {
return 0;
}
while (a > 1) {
q = a / m;
t = m;
m = a % m;
a = t;
t = x0;
x0 = x1 - q * x0;
x1 = t;
}
if (x1 < 0) {
x1 += m0;
}
return x1;
}
// RSA加密
int encrypt(int plaintext, int e, int n) {
int ciphertext = pow(plaintext, e);
ciphertext %= n;
return ciphertext;
}
// RSA解密
int decrypt(int ciphertext, int d, int n) {
int plaintext = pow(ciphertext, d);
plaintext %= n;
return plaintext;
}
int main() {
// 选择素数p和q
int p = 101;
int q = 103;
int n = p * q;
int phi_n = (p - 1) * (q - 1);
// 选择加密指数e
int e = 17;
while (gcd(e, phi_n) != 1) {
e++;
}
// 计算解密指数d
int d = mod_inverse(e, phi_n);
// 显示公钥和私钥
std::cout << "公钥:" << e << ", " << n << std::endl;
std::cout << "私钥:" << d << ", " << n << std::endl;
// 加密和解密测试
int plaintext = 123;
int ciphertext = encrypt(plaintext, e, n);
plaintext = decrypt(ciphertext, d, n);
std::cout << "明文:" << plaintext << std::endl;
std::cout << "密文:" << ciphertext << std::endl;
return 0;
}
```
需要注意的是,本示例代码中的计算过程可能会涉及到大数运算,需要使用高精度库或者使用RSA算法的扩展版本来处理。
相关推荐
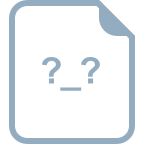
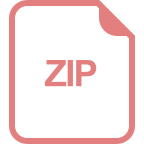














