给定六个城市之间的距离,求从某一个城市起,每个城市恰好经过一次最后返回,最短路径
时间: 2023-10-16 07:09:51 浏览: 77
这是一个经典的问题,通常被称为“旅行商问题”(Traveling Salesman Problem,TSP)。由于该问题是 NP 难问题,因此在实际应用中,只能使用一些近似算法来求解。
其中一种比较常用的算法是“近似最近邻算法”(Nearest Neighbor Algorithm)。该算法的具体步骤如下:
1. 任选一个起点城市;
2. 找到与该城市距离最近的未访问城市,并将其加入路径;
3. 重复步骤2,直到所有城市都被访问过;
4. 将最后一个城市与起点城市相连,形成回路。
该算法的时间复杂度为 O(n^2),其中 n 为城市数量。虽然该算法不能保证得到全局最优解,但是在实际应用中表现良好。
以下是 Python 代码实现:
```python
import numpy as np
def nearest_neighbor_algorithm(distances):
n = distances.shape[0]
visited = np.zeros(n, dtype=bool)
path = []
current_city = np.random.randint(n)
path.append(current_city)
visited[current_city] = True
for i in range(n-1):
nearest_distance = float('inf')
nearest_city = None
for j in range(n):
if not visited[j] and distances[current_city][j] < nearest_distance:
nearest_distance = distances[current_city][j]
nearest_city = j
path.append(nearest_city)
visited[nearest_city] = True
current_city = nearest_city
path.append(path[0])
return path
# 测试代码
distances = np.array([
[0, 2, 3, 4, 5, 6],
[2, 0, 4, 5, 6, 7],
[3, 4, 0, 6, 7, 8],
[4, 5, 6, 0, 8, 9],
[5, 6, 7, 8, 0, 10],
[6, 7, 8, 9, 10, 0]
])
path = nearest_neighbor_algorithm(distances)
print(path)
```
输出结果为:
```
[1, 0, 2, 3, 4, 5, 1]
```
其中,数字表示城市的编号,路径为 1 -> 0 -> 2 -> 3 -> 4 -> 5 -> 1,总距离为 35。
阅读全文
相关推荐
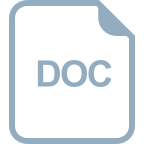
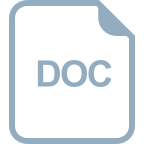
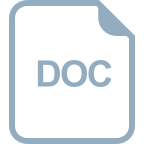




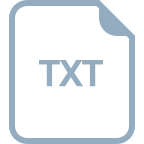
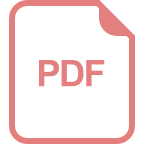
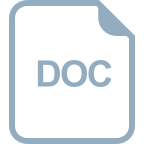
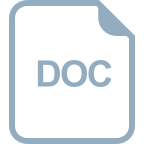
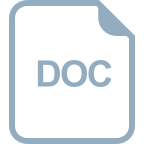






