写一个生产者与消费者的线程问题
时间: 2024-05-07 12:22:13 浏览: 121
生产者与消费者问题是一个经典的线程同步问题,在多线程编程中非常常见。下面是一个简单的生产者与消费者的线程问题的实现。
```python
import threading
import time
import random
# 缓冲区大小
BUFFER_SIZE = 10
# 生产者和消费者的数量
NUM_PRODUCERS = 2
NUM_CONSUMERS = 3
# 缓冲区
buffer = []
# 互斥锁和条件变量
lock = threading.Lock()
not_full = threading.Condition(lock)
not_empty = threading.Condition(lock)
# 定义生产者线程
class Producer(threading.Thread):
def run(self):
global buffer
while True:
# 产生一个随机数作为产品
item = random.randint(1, 100)
# 获取锁
not_full.acquire()
# 如果缓冲区已满,等待消费者消费
while len(buffer) == BUFFER_SIZE:
not_full.wait()
# 将产品加入缓冲区
buffer.append(item)
print("Producer {} produced item {}. Buffer size is {}.".format(self.name, item, len(buffer)))
# 通知消费者缓冲区不为空
not_empty.notify()
# 释放锁
not_full.release()
time.sleep(random.random())
# 定义消费者线程
class Consumer(threading.Thread):
def run(self):
global buffer
while True:
# 获取锁
not_empty.acquire()
# 如果缓冲区为空,等待生产者生产
while len(buffer) == 0:
not_empty.wait()
# 从缓冲区取出产品
item = buffer.pop(0)
print("Consumer {} consumed item {}. Buffer size is {}.".format(self.name, item, len(buffer)))
# 通知生产者缓冲区不满
not_full.notify()
# 释放锁
not_empty.release()
time.sleep(random.random())
# 创建生产者和消费者线程
producers = []
consumers = []
for i in range(NUM_PRODUCERS):
producers.append(Producer())
for i in range(NUM_CONSUMERS):
consumers.append(Consumer())
# 启动线程
for t in producers:
t.start()
for t in consumers:
t.start()
# 等待线程完成
for t in producers:
t.join()
for t in consumers:
t.join()
```
在上面的代码中,我们定义了一个缓冲区作为生产者和消费者之间的交流通道。生产者线程产生随机数作为产品,将产品加入缓冲区;消费者线程从缓冲区取出产品进行消费。生产者和消费者之间需要进行互斥和同步,保证生产者不会往已满的缓冲区中加入产品,消费者不会从空的缓冲区中取出产品。为了实现互斥和同步,我们使用了一个互斥锁和两个条件变量。当缓冲区已满时,生产者线程等待一个条件变量;当缓冲区为空时,消费者线程等待另一个条件变量。当有产品加入或取出缓冲区时,需要通知等待的线程。这样就能够实现生产者和消费者之间的同步。
阅读全文
相关推荐
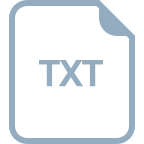
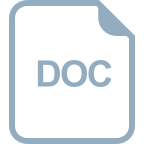
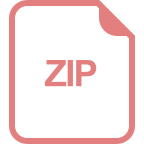
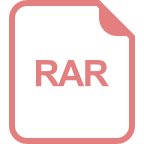
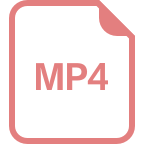
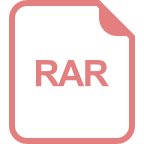
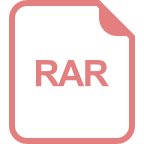
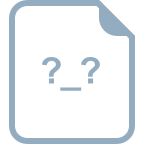
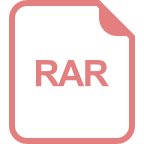
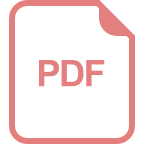