You may notice that entering a non-numeric value and clicking the ‘Check!’ button will cause a run-time error on the console. Therefore, your second task is to improve the application developed in Task 1 to ensure the user is only allowed to supply valid input values, i.e. a 4/5 number between 1 and 10 (inclusive). The application must still function as specified in Task 1 although you may remove the ‘Check!’ button if you wish. Hint: Use another appropriate component in place of the text field.用Java编写
时间: 2024-03-16 13:41:12 浏览: 398
好的,针对您的新问题,我了解了您的需求,下面是使用 Java 编写的代码实现:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class ValidInput extends JFrame implements ActionListener {
private JLabel label;
private JSpinner spinner;
private JButton button;
public ValidInput() {
label = new JLabel("Please enter a number between 1 and 10 (inclusive):");
spinner = new JSpinner(new SpinnerNumberModel(1, 1, 10, 1));
spinner.setPreferredSize(new Dimension(50, 20));
button = new JButton("Submit");
button.addActionListener(this);
JPanel panel = new JPanel();
panel.add(label);
panel.add(spinner);
panel.add(button);
this.add(panel);
this.pack();
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
int input = (int) spinner.getValue();
System.out.println("Valid input received: " + input);
}
public static void main(String[] args) {
new ValidInput();
}
}
```
这段代码使用了 Java Swing 库中的组件来实现用户界面。我们使用了 JLabel 来显示提示信息,使用 JSpinner 来让用户输入数字,使用 JButton 来触发提交操作。
在 JSpinner 中,我们使用了 SpinnerNumberModel 来限制用户只能输入 1 到 10 之间的数字。这样可以保证用户输入的值是合法的。在提交操作中,我们可以直接使用 spinner.getValue() 来获取用户输入的数字,而不需要进行任何解析操作。
通过使用这些组件,我们可以更好地限制用户输入的合法范围,从而避免了在 Task 1 中出现的运行时错误。
阅读全文
相关推荐
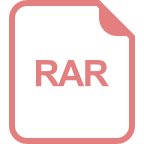
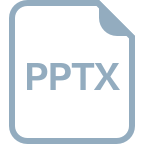


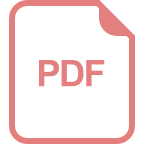
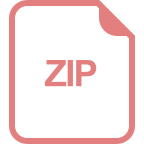








