You may notice that entering a non-numeric value and clicking the ‘Check!’ button will cause a run-time error on the console. Therefore, your second task is to improve the application developed in Task 1 to ensure the user is only allowed to supply valid input values, i.e. a 4/5 number between 1 and 10 (inclusive). The application must still function as specified in Task 1 although you may remove the ‘Check!’ button if you wish.
时间: 2024-03-15 08:44:41 浏览: 71
Based on the requirements of Task 2, the following modifications can be made to the application developed in Task 1:
1. Add input validation to ensure that the user can only input valid values. This can be done by adding an event listener to the input fields that checks the input value against the specified criteria (4/5 number between 1 and 10). If the input value is invalid, display an error message and prevent the user from submitting the form.
2. Remove the 'Check!' button if desired since input validation is being performed dynamically as the user types in the input field.
Here is an example implementation in JavaScript:
```
// Get the input fields
const inputFields = document.querySelectorAll('.input-field');
// Add event listeners to each input field
inputFields.forEach(inputField => {
inputField.addEventListener('input', (event) => {
const inputValue = event.target.value;
const isValid = /^([1-9]|10)(\.\d)?\d?$/.test(inputValue);
const errorElement = inputField.nextElementSibling;
if (!isValid) {
errorElement.innerHTML = 'Invalid input value. Please enter a number between 1 and 10 with up to 1 decimal place.';
inputField.classList.add('invalid');
} else {
errorElement.innerHTML = '';
inputField.classList.remove('invalid');
}
});
});
// Add event listener to the form submission
const form = document.querySelector('form');
form.addEventListener('submit', (event) => {
event.preventDefault();
const inputValues = Array.from(inputFields).map(inputField => inputField.value);
const isValid = inputValues.every(inputValue => /^([1-9]|10)(\.\d)?\d?$/.test(inputValue));
if (isValid) {
const sum = inputValues.reduce((acc, cur) => acc + parseFloat(cur), 0);
const average = sum / inputValues.length;
alert(`Sum: ${sum}\nAverage: ${average.toFixed(2)}`);
} else {
alert('Invalid input values. Please enter numbers between 1 and 10 with up to 1 decimal place.');
}
});
```
In this implementation, the regular expression `/^([1-9]|10)(\.\d)?\d?$/` is used to validate the input values. This regular expression matches any number between 1 and 10 with up to 1 decimal place. The `input` event listener is added to each input field to dynamically validate the input value as the user types. If the input value is invalid, an error message is displayed and the input field is marked as invalid with a red border. If all input values are valid, the form is submitted and the sum and average are calculated and displayed in an alert. If any input value is invalid, an error message is displayed and the form submission is prevented.
阅读全文
相关推荐
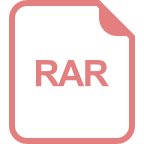
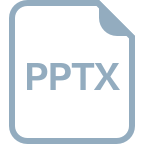

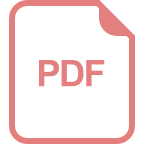
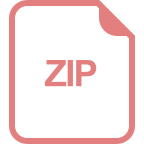









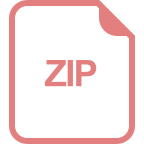
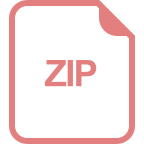
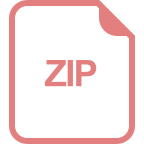
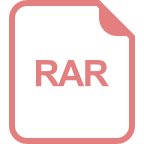