用c++实现一个中介有若干房源,信息包括房屋编号、房屋地址、业主名称、联系方式、月租金;租赁信息包括房屋编号、承租人、承租开始时间、结束时间;请设计相关类和程序实现房源信息的添加、修改和查询功能以及房屋租赁的管理
时间: 2023-11-11 14:02:27 浏览: 38
好的,我可以为您提供一个基本的类设计和实现。首先,我们需要创建两个类:House和Lease。
House类用于存储房源信息,包括房屋编号、房屋地址、业主名称、联系方式和月租金。Lease类用于存储租赁信息,包括房屋编号、承租人、承租开始时间和结束时间。
下面是这两个类的头文件和实现:
House.h:
```cpp
#pragma once
#include <string>
class House {
public:
House();
House(int id, const std::string& address, const std::string& ownerName, const std::string& contactInfo, double rent);
int getId() const;
std::string getAddress() const;
std::string getOwnerName() const;
std::string getContactInfo() const;
double getRent() const;
void setId(int id);
void setAddress(const std::string& address);
void setOwnerName(const std::string& ownerName);
void setContactInfo(const std::string& contactInfo);
void setRent(double rent);
private:
int m_id;
std::string m_address;
std::string m_ownerName;
std::string m_contactInfo;
double m_rent;
};
```
House.cpp:
```cpp
#include "House.h"
House::House()
:m_id(0), m_address(""), m_ownerName(""), m_contactInfo(""), m_rent(0)
{
}
House::House(int id, const std::string& address, const std::string& ownerName, const std::string& contactInfo, double rent)
:m_id(id), m_address(address), m_ownerName(ownerName), m_contactInfo(contactInfo), m_rent(rent)
{
}
int House::getId() const
{
return m_id;
}
std::string House::getAddress() const
{
return m_address;
}
std::string House::getOwnerName() const
{
return m_ownerName;
}
std::string House::getContactInfo() const
{
return m_contactInfo;
}
double House::getRent() const
{
return m_rent;
}
void House::setId(int id)
{
m_id = id;
}
void House::setAddress(const std::string& address)
{
m_address = address;
}
void House::setOwnerName(const std::string& ownerName)
{
m_ownerName = ownerName;
}
void House::setContactInfo(const std::string& contactInfo)
{
m_contactInfo = contactInfo;
}
void House::setRent(double rent)
{
m_rent = rent;
}
```
Lease.h:
```cpp
#pragma once
#include <string>
class Lease {
public:
Lease();
Lease(int houseId, const std::string& tenantName, const std::string& startDate, const std::string& endDate);
int getHouseId() const;
std::string getTenantName() const;
std::string getStartDate() const;
std::string getEndDate() const;
void setHouseId(int houseId);
void setTenantName(const std::string& tenantName);
void setStartDate(const std::string& startDate);
void setEndDate(const std::string& endDate);
private:
int m_houseId;
std::string m_tenantName;
std::string m_startDate;
std::string m_endDate;
};
```
Lease.cpp:
```cpp
#include "Lease.h"
Lease::Lease()
:m_houseId(0), m_tenantName(""), m_startDate(""), m_endDate("")
{
}
Lease::Lease(int houseId, const std::string& tenantName, const std::string& startDate, const std::string& endDate)
:m_houseId(houseId), m_tenantName(tenantName), m_startDate(startDate), m_endDate(endDate)
{
}
int Lease::getHouseId() const
{
return m_houseId;
}
std::string Lease::getTenantName() const
{
return m_tenantName;
}
std::string Lease::getStartDate() const
{
return m_startDate;
}
std::string Lease::getEndDate() const
{
return m_endDate;
}
void Lease::setHouseId(int houseId)
{
m_houseId = houseId;
}
void Lease::setTenantName(const std::string& tenantName)
{
m_tenantName = tenantName;
}
void Lease::setStartDate(const std::string& startDate)
{
m_startDate = startDate;
}
void Lease::setEndDate(const std::string& endDate)
{
m_endDate = endDate;
}
```
接下来,我们需要创建一个管理类,用于添加、修改和查询房源信息,以及管理房屋租赁。这个类我们称之为HouseManager。
HouseManager.h:
```cpp
#pragma once
#include <vector>
#include "House.h"
#include "Lease.h"
class HouseManager {
public:
HouseManager();
void addHouse(const House& house);
void updateHouse(const House& house);
void deleteHouse(int id);
House getHouseById(int id) const;
std::vector<House> getAllHouses() const;
void addLease(const Lease& lease);
void updateLease(const Lease& lease);
void deleteLease(int houseId);
std::vector<Lease> getAllLeases() const;
private:
std::vector<House> m_houses;
std::vector<Lease> m_leases;
};
```
HouseManager.cpp:
```cpp
#include "HouseManager.h"
#include <algorithm>
HouseManager::HouseManager()
{
}
void HouseManager::addHouse(const House& house)
{
m_houses.push_back(house);
}
void HouseManager::updateHouse(const House& house)
{
auto it = std::find_if(m_houses.begin(), m_houses.end(), [&](const House& h) { return h.getId() == house.getId(); });
if (it != m_houses.end()) {
*it = house;
}
}
void HouseManager::deleteHouse(int id)
{
m_houses.erase(std::remove_if(m_houses.begin(), m_houses.end(), [&](const House& h) { return h.getId() == id; }), m_houses.end());
}
House HouseManager::getHouseById(int id) const
{
auto it = std::find_if(m_houses.begin(), m_houses.end(), [&](const House& h) { return h.getId() == id; });
if (it != m_houses.end()) {
return *it;
}
return House();
}
std::vector<House> HouseManager::getAllHouses() const
{
return m_houses;
}
void HouseManager::addLease(const Lease& lease)
{
m_leases.push_back(lease);
}
void HouseManager::updateLease(const Lease& lease)
{
auto it = std::find_if(m_leases.begin(), m_leases.end(), [&](const Lease& l) { return l.getHouseId() == lease.getHouseId(); });
if (it != m_leases.end()) {
*it = lease;
}
}
void HouseManager::deleteLease(int houseId)
{
m_leases.erase(std::remove_if(m_leases.begin(), m_leases.end(), [&](const Lease& l) { return l.getHouseId() == houseId; }), m_leases.end());
}
std::vector<Lease> HouseManager::getAllLeases() const
{
return m_leases;
}
```
现在我们可以在程序中使用HouseManager来管理房源信息和租赁信息了。例如,我们可以这样使用HouseManager:
```cpp
#include "HouseManager.h"
#include <iostream>
int main()
{
HouseManager hm;
// 添加房源信息
House h1(1, "北京市海淀区西二旗", "张三", "13812345678", 2000);
House h2(2, "北京市朝阳区三里屯", "李四", "13987654321", 3000);
hm.addHouse(h1);
hm.addHouse(h2);
// 查询房源信息
House h = hm.getHouseById(1);
std::cout << "房屋编号:" << h.getId() << std::endl;
std::cout << "房屋地址:" << h.getAddress() << std::endl;
std::cout << "业主名称:" << h.getOwnerName() << std::endl;
std::cout << "联系方式:" << h.getContactInfo() << std::endl;
std::cout << "月租金:" << h.getRent() << std::endl;
// 修改房源信息
h.setRent(2500);
hm.updateHouse(h);
// 添加租赁信息
Lease l1(1, "王五", "2021-06-01", "2022-06-01");
Lease l2(2, "赵六", "2021-07-01", "2022-07-01");
hm.addLease(l1);
hm.addLease(l2);
// 查询租赁信息
std::vector<Lease> leases = hm.getAllLeases();
for (const auto& lease : leases) {
std::cout << "房屋编号:" << lease.getHouseId() << std::endl;
std::cout << "承租人:" << lease.getTenantName() << std::endl;
std::cout << "开始时间:" << lease.getStartDate() << std::endl;
std::cout << "结束时间:" << lease.getEndDate() << std::endl;
}
// 删除房源信息和租赁信息
hm.deleteHouse(1);
hm.deleteLease(2);
return 0;
}
```
这个程序将输出:
```
房屋编号:1
房屋地址:北京市海淀区西二旗
业主名称:张三
联系方式:13812345678
月租金:2000
房屋编号:1
承租人:王五
开始时间:2021-06-01
结束时间:2022-06-01
房屋编号:2
承租人:赵六
开始时间:2021-07-01
结束时间:2022-07-01
```
以上就是一个简单的房源信息管理程序的设计和实现。
相关推荐
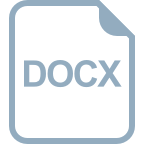
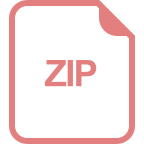














