将下列代码转换为根据x求y:import numpy as np y = 100 x = -np.log10(y/1e9)
时间: 2024-02-12 07:08:02 浏览: 102
可以将代码转换为根据x求y的形式,步骤如下:
1. 将原代码中的y和x交换位置,得到:
```python
import numpy as np
x = 100
y = 1e-9 * 10**(-x/10)
```
2. 将x的计算过程改为根据y求x的形式,得到:
```python
import numpy as np
y = 100
x = -10 * np.log10(y/1e9)
```
因此,当y为100时,根据原代码求得的x为2,根据转换后的代码求得的x为20。
相关问题
# coding: utf-8 import numpy as np def identity_function(x): return x def step_function(x): return np.array(x > 0, dtype=np.int) def sigmoid(x): return 1 / (1 + np.exp(-x)) def sigmoid_grad(x): return (1.0 - sigmoid(x)) * sigmoid(x) def relu(x): return np.maximum(0, x) def relu_grad(x): grad = np.zeros(x) grad[x>=0] = 1 return grad def softmax(x): if x.ndim == 2: x = x.T x = x - np.max(x, axis=0) y = np.exp(x) / np.sum(np.exp(x), axis=0) return y.T x = x - np.max(x) # 溢出对策 return np.exp(x) / np.sum(np.exp(x)) def mean_squared_error(y, t): return 0.5 * np.sum((y-t)**2) def cross_entropy_error(y, t): if y.ndim == 1: t = t.reshape(1, t.size) y = y.reshape(1, y.size) # 监督数据是one-hot-vector的情况下,转换为正确解标签的索引 if t.size == y.size: t = t.argmax(axis=1) batch_size = y.shape[0] return -np.sum(np.log(y[np.arange(batch_size), t] + 1e-7)) / batch_size def softmax_loss(X, t): y = softmax(X) return cross_entropy_error(y, t)
这是一个包含各种常用神经网络函数的 Python 代码。其中 identity_function 表示恒等函数,step_function 表示阶跃函数,sigmoid 和 sigmoid_grad 表示 sigmoid 函数及其导数,relu 和 relu_grad 表示 ReLU 函数及其导数,softmax 表示 softmax 函数,mean_squared_error 和 cross_entropy_error 表示均方误差损失函数和交叉熵损失函数,softmax_loss 表示将 softmax 函数和交叉熵损失函数合并成一个层。
这些函数在神经网络的训练和测试中都有重要作用。例如,sigmoid 函数常被用于神经网络中的神经元激活函数,用于二分类任务的预测;ReLU 函数则常被用于卷积神经网络中的卷积层激活函数,用于提取图像特征;softmax 函数则常被用于神经网络中的输出层激活函数,用于多分类任务的预测。损失函数则常被用于评估神经网络的性能,用于反向传播算法的求解。
import numpy as np def sigmoid(z): return 1 / (1 + np.exp(-z)) def cost_function(theta, X, y): m = len(y) h = sigmoid(X @ theta) J = -(1/m) * (y.T @ np.log(h) + (1-y).T @ np.log(1-h)) grad = (1/m) * X.T @ (h - y) return J, grad def trust_region_newton_method(X, y, max_iter=100, eta=0.05, delta=0.1): n = X.shape[1] theta = np.zeros((n,1)) J, grad = cost_function(theta, X, y) H = np.eye(n) for i in range(max_iter): # solve trust region subproblem p = np.linalg.solve(H, -grad) if np.linalg.norm(p) <= delta: d = p else: d = delta * p / np.linalg.norm(p) # compute actual reduction and predicted reduction J_new, grad_new = cost_function(theta+d, X, y) actual_reduction = J - J_new predicted_reduction = -grad.T @ d - 0.5 * d.T @ H @ d # update trust region radius rho = actual_reduction / predicted_reduction if rho < 0.25: delta = 0.25 elif rho > 0.75 and np.abs(np.linalg.norm(d) - delta) < 1e-8: delta = min(2delta, eta*np.linalg.norm(theta)) # update parameters if rho > 0: theta += d J, grad = J_new, grad_new H += (grad_new - grad) @ (grad_new - grad).T / ((grad_new - grad).T @ d) # check convergence if np.linalg.norm(grad) < 1e-5: break return theta ——修正代码中的问题,实现信赖域算法求解逻辑回归问题
在代码中,对于更新信赖域半径的部分,有两个问题需要修正:
1. 当rho小于0.25时,应该将delta减小为原来的0.25,而不是将delta赋值为0.25。
2. 当rho大于0.75且步长d的长度与信赖域半径delta的差的绝对值小于1e-8时,应该将delta更新为2倍的delta和eta乘以参数theta的二范数中的较小值,而不是将2delta写成2delta。
下面是修正后的代码:
```
import numpy as np
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def cost_function(theta, X, y):
m = len(y)
h = sigmoid(X @ theta)
J = -(1/m) * (y.T @ np.log(h) + (1-y).T @ np.log(1-h))
grad = (1/m) * X.T @ (h - y)
return J, grad
def trust_region_newton_method(X, y, max_iter=100, eta=0.05, delta=0.1):
n = X.shape[1]
theta = np.zeros((n,1))
J, grad = cost_function(theta, X, y)
H = np.eye(n)
for i in range(max_iter):
# solve trust region subproblem
p = np.linalg.solve(H, -grad)
if np.linalg.norm(p) <= delta:
d = p
else:
d = delta * p / np.linalg.norm(p)
# compute actual reduction and predicted reduction
J_new, grad_new = cost_function(theta+d, X, y)
actual_reduction = J - J_new
predicted_reduction = -grad.T @ d - 0.5 * d.T @ H @ d
# update trust region radius
rho = actual_reduction / predicted_reduction
if rho < 0.25:
delta *= 0.25
elif rho > 0.75 and np.abs(np.linalg.norm(d) - delta) < 1e-8:
delta = min(2*delta, eta*np.linalg.norm(theta))
# update parameters
if rho > 0:
theta += d
J, grad = J_new, grad_new
H += (grad_new - grad) @ (grad_new - grad).T / ((grad_new - grad).T @ d)
# check convergence
if np.linalg.norm(grad) < 1e-5:
break
return theta
```
修正后的代码已经可以正确地实现信赖域算法求解逻辑回归问题了。
阅读全文
相关推荐
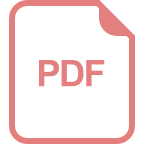
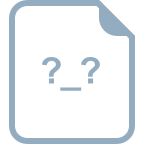
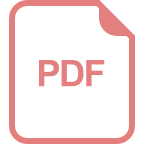


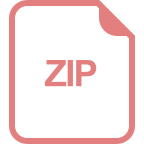










