调用chatgpt api与数据库进行交互
时间: 2023-10-20 12:05:18 浏览: 45
首先,你需要确定使用哪个 ChatGPT API,以及你的数据库类型。如果你使用的是 Python 编程语言,可以考虑使用以下库:
- `openai`:OpenAI 公司开发的 Python 库,提供了与 GPT 系列模型交互的功能。
- `transformers`:Hugging Face 公司开发的 Python 库,提供了与多种 GPT 系列模型交互的功能。
- `pymongo`:MongoDB 官方提供的 Python 库,用于连接和操作 MongoDB 数据库。
以下是一个使用 OpenAI 的 ChatGPT API 和 MongoDB 数据库进行交互的示例代码:
```python
import openai
import pymongo
# 连接 MongoDB 数据库
client = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["mydatabase"]
collection = db["mycollection"]
# 连接 OpenAI API
openai.api_key = "YOUR_API_KEY"
model_engine = "text-davinci-002" # ChatGPT 模型名称
# ChatGPT 对话函数
def chat_with_gpt(prompt):
response = openai.Completion.create(
engine=model_engine,
prompt=prompt,
max_tokens=1024,
n=1,
stop=None,
temperature=0.5,
)
return response.choices[0].text.strip()
# 对话交互
while True:
user_input = input("你:")
if user_input == "退出":
break
chat_prompt = collection.find_one({"user_input": user_input})
if chat_prompt:
chat_response = chat_prompt["chat_response"]
else:
chat_response = chat_with_gpt(user_input)
collection.insert_one({"user_input": user_input, "chat_response": chat_response})
print("AI:", chat_response)
```
在这个示例代码中,我们使用了 OpenAI 的 `text-davinci-002` 模型作为 ChatGPT 模型,并使用了 MongoDB 数据库来保存用户输入和 AI 回复的记录。在对话交互过程中,如果用户输入已经出现过,我们会直接从数据库中获取 AI 的回复,否则使用 ChatGPT API 生成回复,并将用户输入和 AI 回复记录到数据库中。
相关推荐
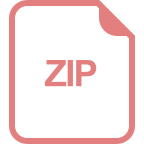
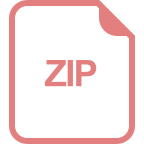










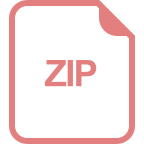
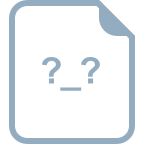
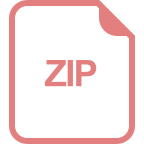
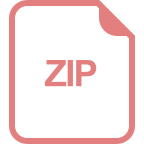