sakai soap 获得某站点所有学生的成绩的python代码
时间: 2024-03-02 16:41:19 浏览: 90
要通过Sakai SOAP API获取某站点所有学生的成绩,可以使用下面的 Python 代码:
```python
import requests
import xml.etree.ElementTree as ET
# 设置Sakai SOAP API的URL和访问令牌
url = "http://your-sakai-server/sakai-ws/soap/sakai?wsdl"
token = "your-access-token"
# 设置SOAP消息体
body = """
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:api="http://api.sakai.org">
<soapenv:Header>
<api:sessionid>{}</api:sessionid>
</soapenv:Header>
<soapenv:Body>
<api:getSiteStudentsWithRole>
<siteId>site-id</siteId>
<role>Student</role>
</api:getSiteStudentsWithRole>
</soapenv:Body>
</soapenv:Envelope>
""".format(token)
# 发送SOAP请求并解析响应
response = requests.post(url, data=body)
root = ET.fromstring(response.content)
# 解析学生列表,并获取每个学生的成绩
students = root.find(".//{http://api.sakai.org}students")
for student in students:
username = student.find("{http://api.sakai.org}userId").text
grades_body = """
<soapenv:Envelope xmlns:soapenv="http://schemas.xmlsoap.org/soap/envelope/" xmlns:api="http://api.sakai.org">
<soapenv:Header>
<api:sessionid>{}</api:sessionid>
</soapenv:Header>
<soapenv:Body>
<api:getGradebookForUser>
<siteId>site-id</siteId>
<userId>{}</userId>
</api:getGradebookForUser>
</soapenv:Body>
</soapenv:Envelope>
""".format(token, username)
grades_response = requests.post(url, data=grades_body)
grades_root = ET.fromstring(grades_response.content)
grades = grades_root.find(".//{http://api.sakai.org}grade")
if grades is not None:
for grade in grades:
item_name = grade.find("{http://api.sakai.org}name").text
score = grade.find("{http://api.sakai.org}score").text
print("学生{}的{}成绩为{}".format(username, item_name, score))
```
请注意,代码中的 `your-sakai-server`、`your-access-token` 和 `site-id` 都需要替换为实际的值。此外,此代码使用了Python标准库中的 `requests` 和 `xml.etree.ElementTree` 模块,因此请确保它们已经安装好。
阅读全文
相关推荐
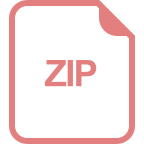















