ffmpeg4.4.4 mp4转h264 visual studio2022 cpp中文示例
时间: 2024-03-20 07:32:17 浏览: 12
下面是一个简单的示例代码,可以在 Visual Studio 2022 中使用 FFmpeg 4.4.4 将 MP4 文件转换为 H.264 编码的视频:
```cpp
#include <iostream>
#include <string>
#include <fstream>
extern "C" {
#include <libavformat/avformat.h>
#include <libavcodec/avcodec.h>
#include <libswscale/swscale.h>
}
int main() {
// 初始化 FFmpeg
av_register_all();
avcodec_register_all();
// 打开输入文件
std::string input_filename = "input.mp4";
AVFormatContext* input_format_context = NULL;
if (avformat_open_input(&input_format_context, input_filename.c_str(), NULL, NULL) != 0) {
std::cerr << "Failed to open input file." << std::endl;
return 1;
}
// 查找视频流
int video_stream_index = -1;
for (unsigned int i = 0; i < input_format_context->nb_streams; i++) {
if (input_format_context->streams[i]->codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
video_stream_index = i;
break;
}
}
if (video_stream_index == -1) {
std::cerr << "Failed to find video stream." << std::endl;
return 1;
}
// 获取输入视频流的编解码器参数
AVCodecParameters* input_codec_params = input_format_context->streams[video_stream_index]->codecpar;
// 查找编解码器
AVCodec* codec = avcodec_find_decoder(input_codec_params->codec_id);
if (codec == NULL) {
std::cerr << "Failed to find codec." << std::endl;
return 1;
}
// 初始化编解码器上下文
AVCodecContext* codec_context = avcodec_alloc_context3(codec);
if (avcodec_parameters_to_context(codec_context, input_codec_params) != 0) {
std::cerr << "Failed to copy codec parameters to codec context." << std::endl;
return 1;
}
if (avcodec_open2(codec_context, codec, NULL) != 0) {
std::cerr << "Failed to open codec." << std::endl;
return 1;
}
// 打开输出文件
std::string output_filename = "output.h264";
std::ofstream output_file(output_filename, std::ios::binary);
// 初始化输出视频帧
AVFrame* frame = av_frame_alloc();
if (frame == NULL) {
std::cerr << "Failed to allocate frame." << std::endl;
return 1;
}
// 初始化转换器
SwsContext* sws_context = sws_getContext(codec_context->width, codec_context->height, codec_context->pix_fmt, codec_context->width, codec_context->height, AV_PIX_FMT_YUV420P, SWS_BICUBIC, NULL, NULL, NULL);
if (sws_context == NULL) {
std::cerr << "Failed to initialize converter." << std::endl;
return 1;
}
// 读取并转换视频帧
AVPacket packet;
while (av_read_frame(input_format_context, &packet) == 0) {
if (packet.stream_index == video_stream_index) {
int frame_finished = 0;
avcodec_decode_video2(codec_context, frame, &frame_finished, &packet);
if (frame_finished) {
sws_scale(sws_context, frame->data, frame->linesize, 0, codec_context->height, frame->data, frame->linesize);
output_file.write(reinterpret_cast<const char*>(frame->data[0]), codec_context->width * codec_context->height);
output_file.write(reinterpret_cast<const char*>(frame->data[1]), codec_context->width * codec_context->height / 4);
output_file.write(reinterpret_cast<const char*>(frame->data[2]), codec_context->width * codec_context->height / 4);
}
}
av_free_packet(&packet);
}
// 清理
av_frame_free(&frame);
sws_freeContext(sws_context);
avcodec_close(codec_context);
avformat_close_input(&input_format_context);
output_file.close();
return 0;
}
```
这个示例代码假设输入文件的视频流使用 H.264 编码,输出文件将包含 H.264 编码的视频数据。如果输入文件使用其他编码格式,需要修改代码以使用正确的编解码器。另外,本示例代码并未处理音频流,如果需要转换音频流,请参考 FFmpeg 的官方文档。
相关推荐
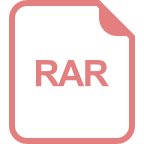
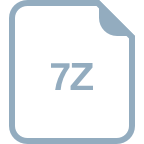














