python迷宫地图代码_用Python代码来解图片迷宫的方法整理
时间: 2024-05-13 22:19:45 浏览: 173
以下是一个简单的 Python 代码示例,可以用来解决迷宫地图问题:
```python
from PIL import Image
# 读取图片
img = Image.open('maze.png')
width, height = img.size
# 将像素点转换成 0/1 的二维数组
maze = []
for y in range(height):
row = []
for x in range(width):
r, g, b = img.getpixel((x, y))
if r == 255 and g == 255 and b == 255:
row.append(1) # 白色像素表示可通行的路
else:
row.append(0) # 其他颜色表示墙
maze.append(row)
# 定义起点和终点
start = (1, 1)
end = (width-2, height-2)
# 定义移动方向
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)]
# 定义 BFS 算法
def bfs(maze, start, end):
queue = [start]
visited = set()
while queue:
x, y = queue.pop(0)
if (x, y) == end:
return True
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < width and 0 <= ny < height and maze[ny][nx] == 1 and (nx, ny) not in visited:
queue.append((nx, ny))
visited.add((nx, ny))
maze[ny][nx] = maze[y][x] + 1 # 记录到起点的距离
return False
# 运行 BFS 算法
if bfs(maze, start, end):
print('迷宫有解')
# 回溯路径
path = [end]
x, y = end
while (x, y) != start:
for dx, dy in directions:
nx, ny = x + dx, y + dy
if 0 <= nx < width and 0 <= ny < height and maze[ny][nx] == maze[y][x] - 1:
path.append((nx, ny))
x, y = nx, ny
break
path.append(start)
path.reverse()
print('路径为', path)
else:
print('迷宫无解')
```
这段代码使用了 PIL 库来读取迷宫图片,并将像素点转换成一个二维数组。然后使用 BFS 算法来搜索从起点到终点的最短路径,并记录下路径。最后输出路径即可。
阅读全文
相关推荐
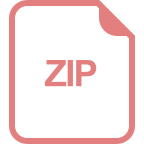
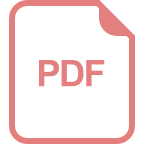

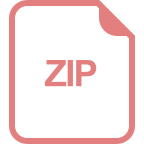
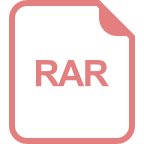




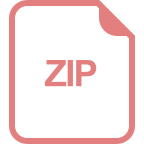
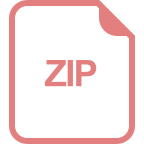
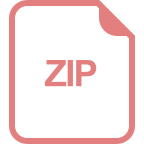
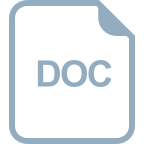
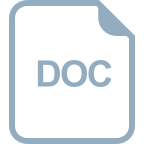


